customizable_date_picker 0.0.3
customizable_date_picker: ^0.0.3 copied to clipboard
A Customizable_date_picker
customizable_date_picker #
A Customizable_date_picker
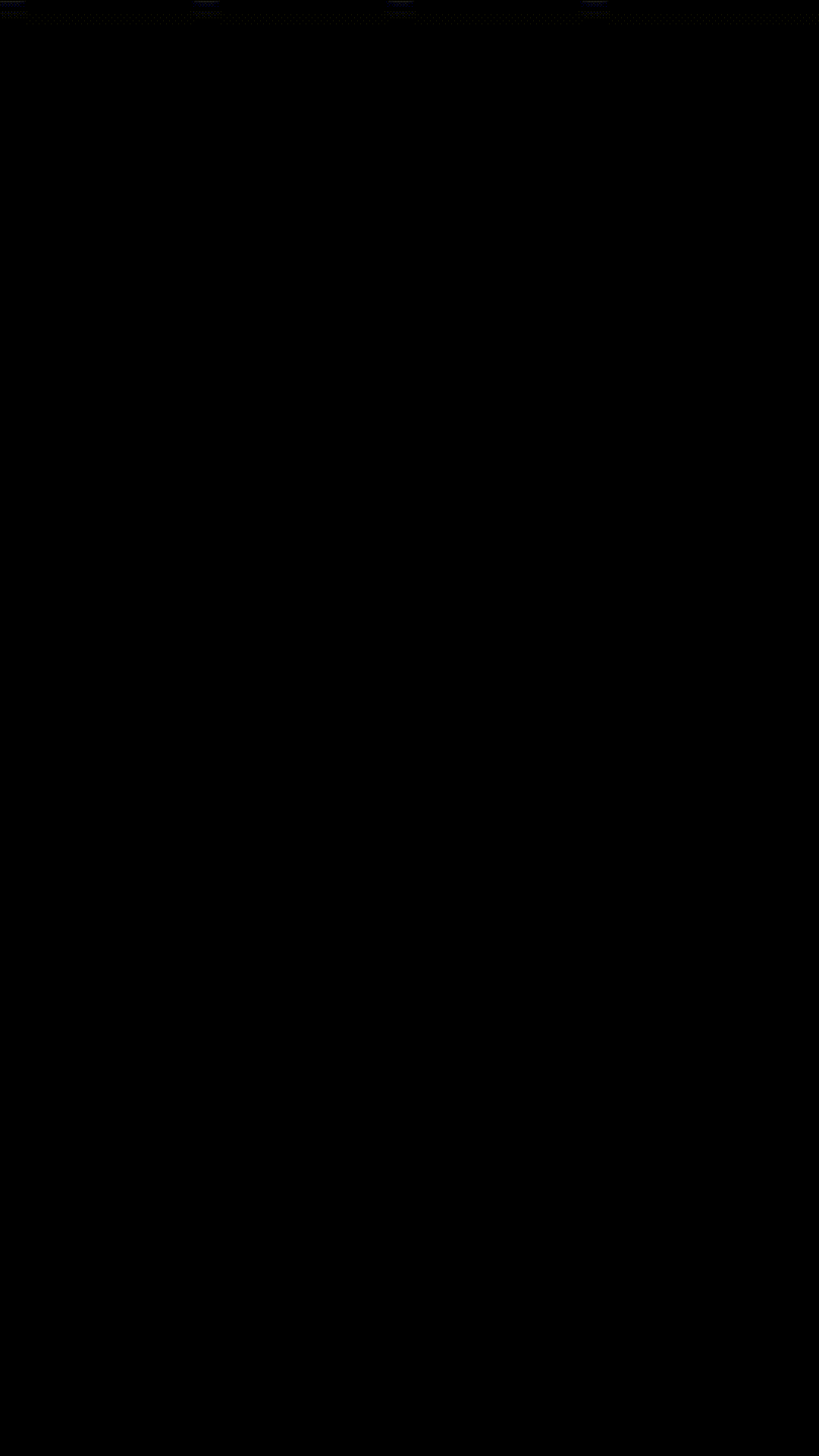
Features #
- Fully Customizable with Custom Builders.
- Can Attach dynamic Data to each day.
- Default Version, Ready to Use.
Getting started #
In the pubspec.yaml
of your flutter project, add the following dependency:
dependencies:
...
customizable_date_picker: "^0.0.3"
In your library add the following import:
import 'package:customizable_date_picker/customizable_date_picker.dart';
For help getting started with Flutter, view the online documentation.
Attributes #
CustomDatePicker #
attribute | type | description |
---|---|---|
controller | CustomDatePickerController | Widget controller |
activeDayTextStyle | TextStyle | normal day color |
hiddenDayTextStyle | TextStyle | hidden day color |
headerTextStyle | TextStyle | TextStyle of days abbreviations |
selectedDaysColor | Color | color of selected day |
headerBackgroundColor | Color | Color of the Header |
daysOfWeek | List | Days abbreviations |
background | Widget | Background widget,could be anything, Image,Animations,Colors... |
dayItemBuilder | Widget Function(CustomDay day, int index) | day item builder |
monthTitleBuilder | Widget Function(DateTime date) | month Title Item Builder |
Warning:
One you chose to use a builder, most other styling attributes will be ignored.
CustomDatePickerController #
attribute | type | description |
---|---|---|
start | DateTime | first month in the calendar |
end | DateTime | last month in the calendar |
generateCustomDay | CustomDay Function(DateTime firstDate, DateTime lastDate) | generate dynamic for each Day |
Simple Use #
CustomDatePickerController _controller = new CustomDatePickerController();
@override
Widget build(BuildContext context) {
return Container(
child: Padding(
padding: const EdgeInsets.all(25.0),
child: Card(
child: CustomDatePicker(
_controller
),
),
),
);
}
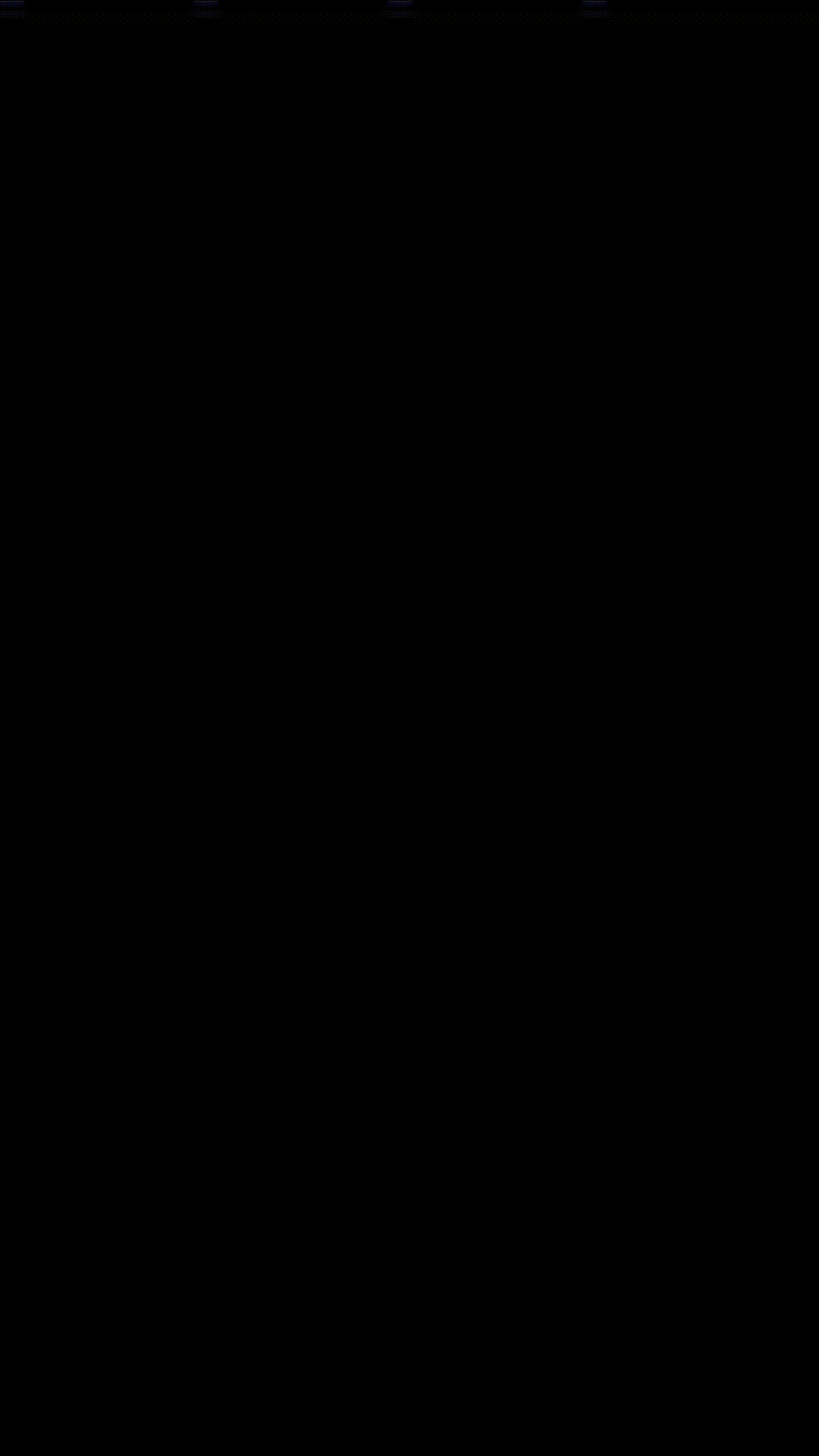
You can find the complete example in the Example project.
Using Custom Builder #
You can specify how every part of the Widget should be built:
Widget build(BuildContext context) {
return Container(
child: Padding(
padding: const EdgeInsets.all(25.0),
child: Card(
child: CustomDatePicker(
_controller,
dayItemBuilder: dayItemBuilder,
monthTitleBuilder: monthTitleBuilder,
headerBackgroundColor: Colors.red,
headerTextStyle: TextStyle(color: Colors.white,fontWeight: FontWeight.w700),
),
),
),
);
}
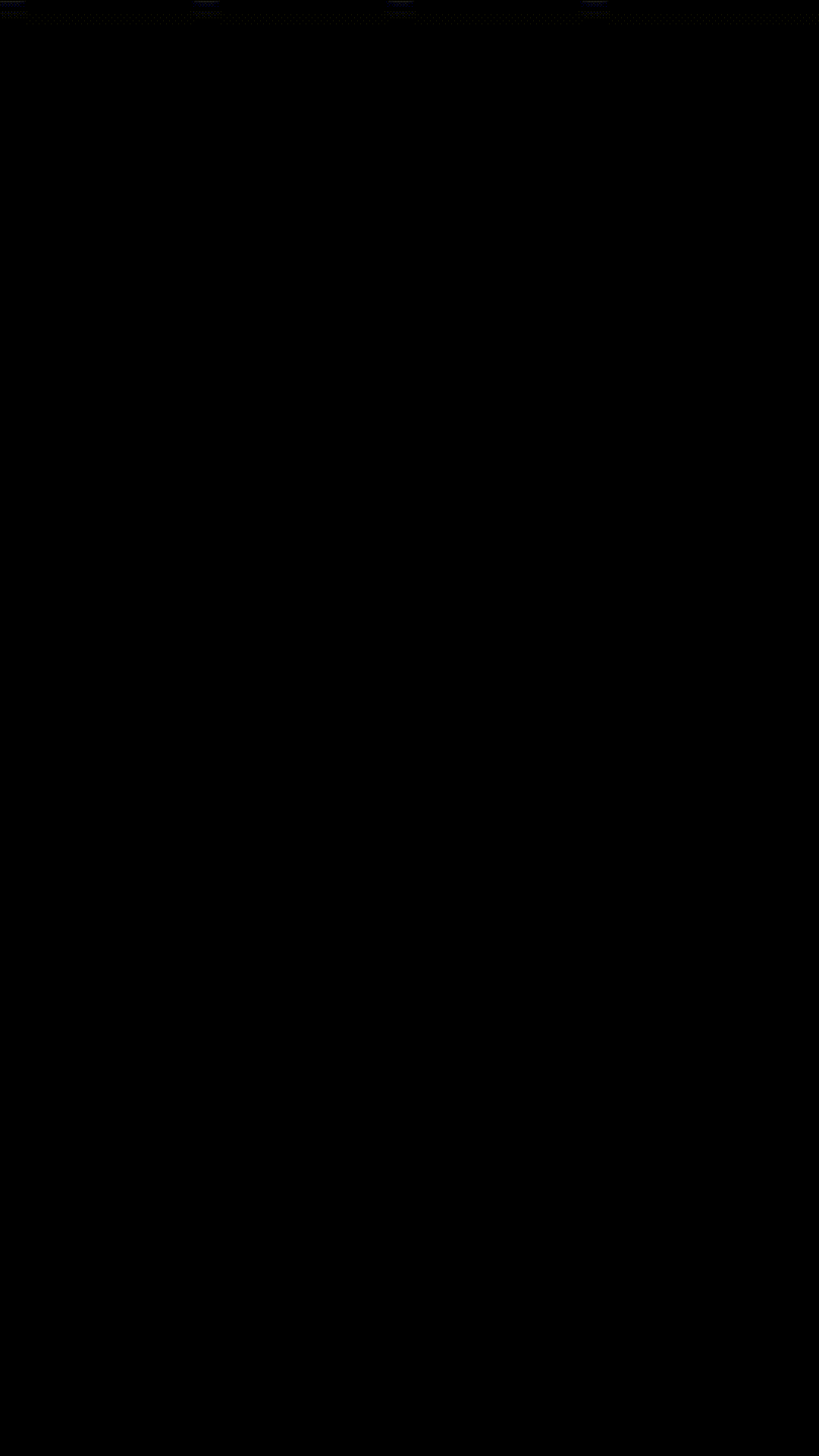
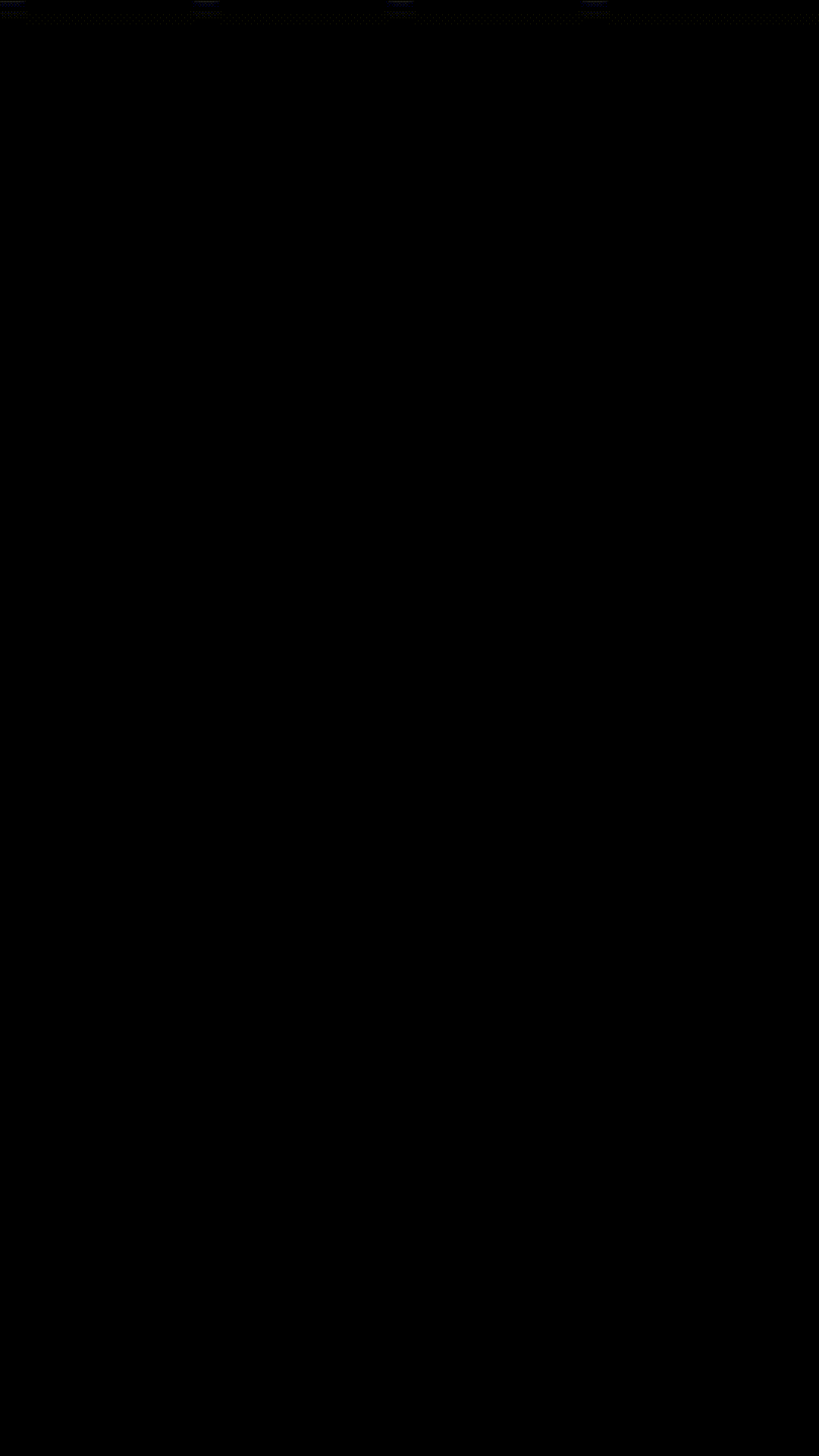
You can find the complete example in the Example project.
PopUp using AlertDialog #
void _showPopUp() {
showDialog(
context: context,
builder: (BuildContext context) {
// return object of type Dialog
return AlertDialog(
title: new Text("Date Picker"),
content: Card(
child: CustomDatePicker(
_controller,
dayItemBuilder: dayItemBuilder,
monthTitleBuilder: monthTitleBuilder,
headerBackgroundColor: Colors.black,
headerTextStyle: TextStyle(color: Colors.white,fontWeight: FontWeight.w700),
),
),
actions: <Widget>[
// usually buttons at the bottom of the dialog
new FlatButton(
child: new Text("Close"),
onPressed: () {
Navigator.of(context).pop();
},
),
new FlatButton(
child: new Text("Okay"),
onPressed: () {
Navigator.of(context).pop();
},
),
],
);
},
);
}
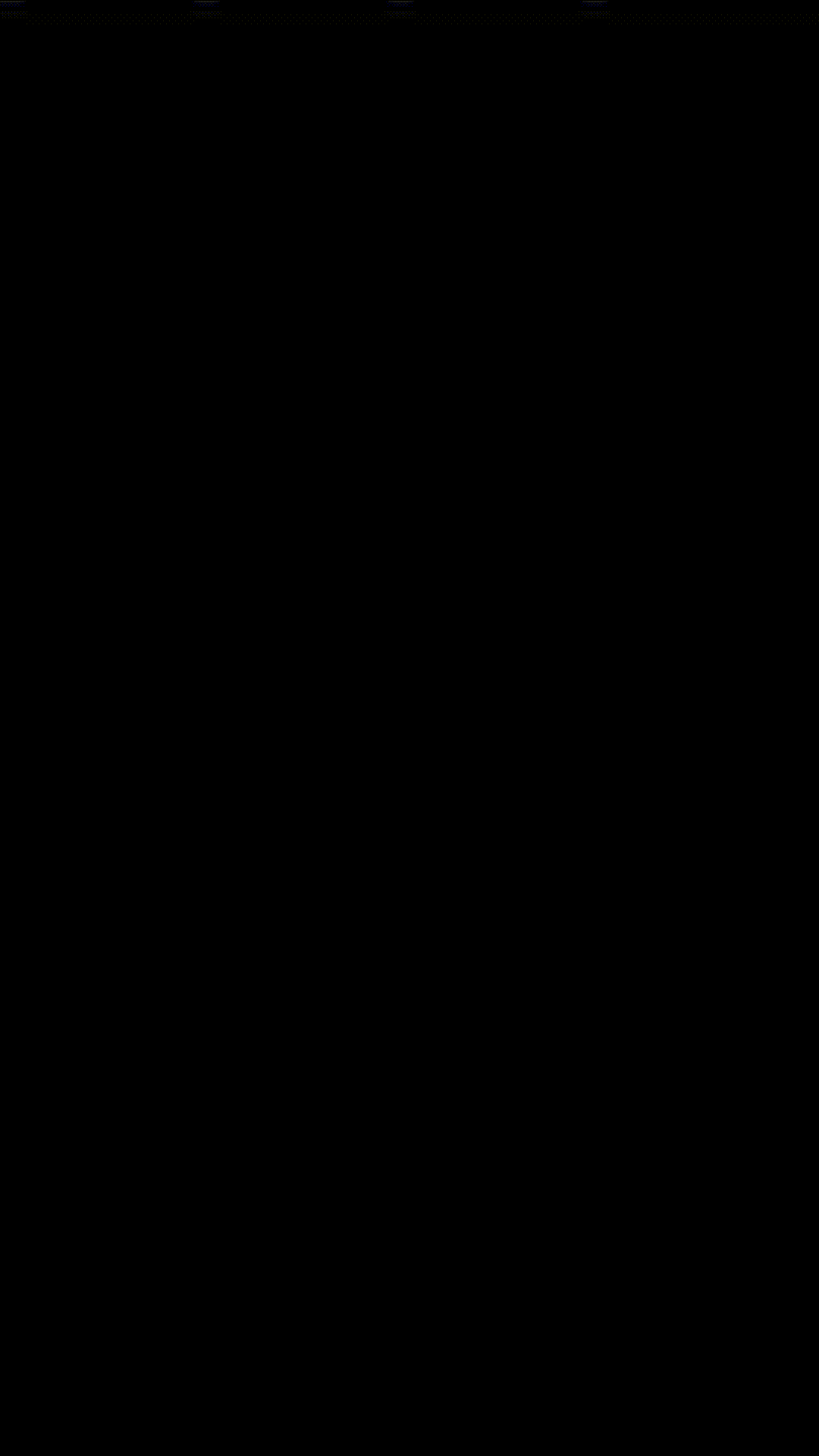
You can find the complete example in the Example project.
Using dynamic Data #
You can use generateCustomDay to initialize CustomDay Objects with dynamic data.
CustomDatePickerController _controller = new CustomDatePickerController(
start: DateTime.now().add(Duration(days: -300)),
end: DateTime.now().add(Duration(days: 300)),
generateCustomDay: generateDay
);
static CustomDay generateDay(DateTime dateTime){
Random random = new Random();
int randomNumber = random.nextInt(200)+70;
int state = random.nextInt(3)*random.nextInt(2)*random.nextInt(2);
return CustomDay(dateTime, {"cost":randomNumber,"state":state});
}
You can also set Data using
controler.setDayData(DateTime date, dynamic data)
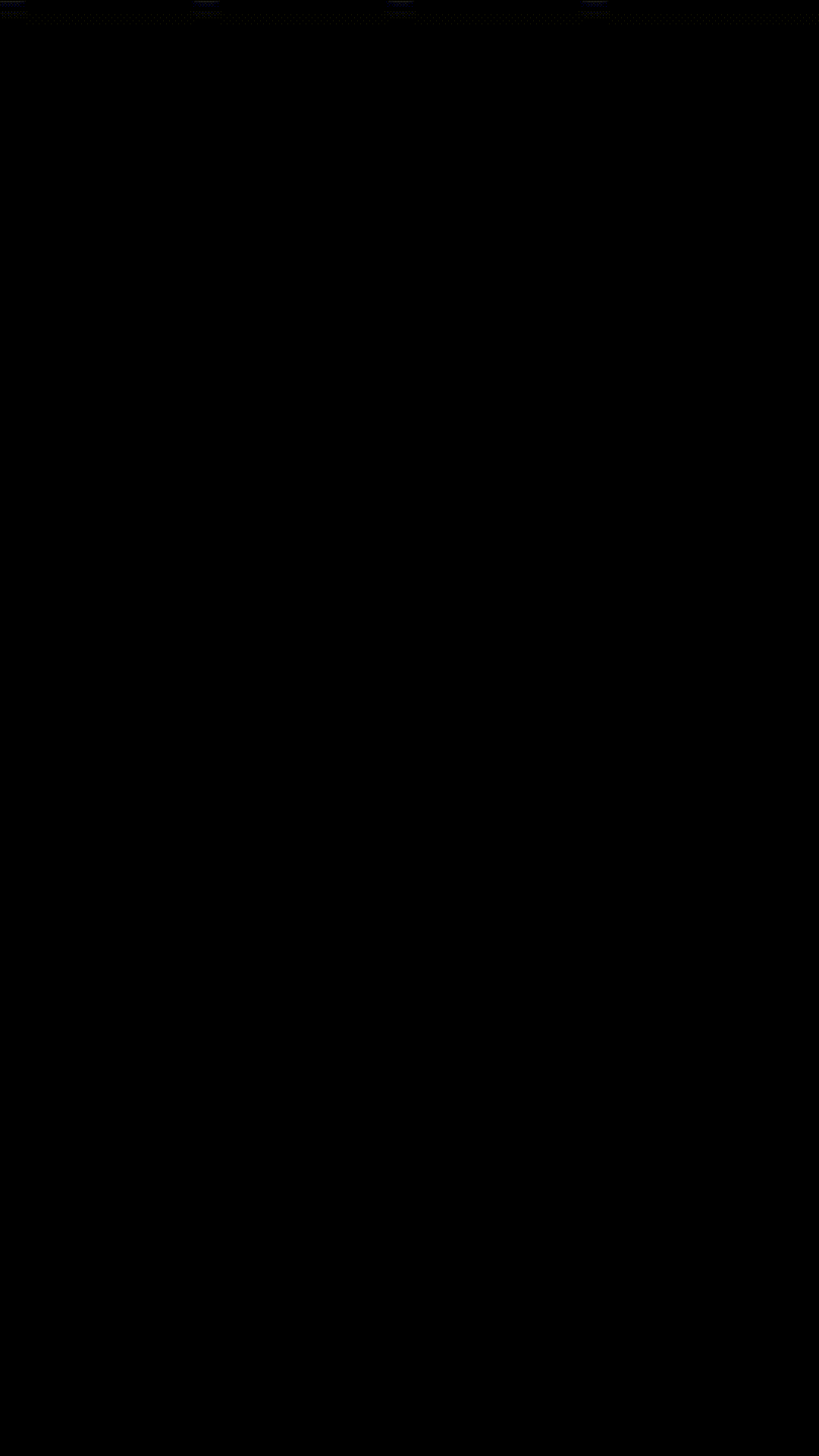
You can find the complete example in the Example project.
Changelog #
Please see the Changelog page to know what's recently changed.
Contributions #
Feel free to contribute to this project.
If you find a bug or want a feature, but don't know how to fix/implement it, please fill an issue.
If you fixed a bug or implemented a new feature, please send a pull request.