connectivity_wrapper 1.2.7
connectivity_wrapper: ^1.2.7 copied to clipboard
A new Flutter package which provides network-aware widgets.It allows Flutter apps provide feedback on your app when it's not connected to it, or when there's no connection.
NonStop
Digital Product Development Experts for Startups & Enterprises
connectivity_wrapper #
This plugin allows Flutter apps provide feedback on your app when it's not connected to it, or when there's no connection.
Requirements #
- Flutter >=3.19.0
- Dart >=3.3.0 <4.0.0
- iOS >=12.0
- MacOS >=10.14
- Android
compileSDK
34 - Java 17
- Android Gradle Plugin >=8.3.0
- Gradle wrapper >=8.4
Usage #
import 'package:connectivity_wrapper/connectivity_wrapper.dart';
Check if device is connected to internet or not
...
onTap: () async {
if (await ConnectivityWrapper.instance.isConnected) {
showSnackBar(
_scaffoldKey,
title: "You Are Connected",
color: Colors.green,
);
} else {
showSnackBar(
_scaffoldKey,
title: "You Are Not Connected",
);
}
},
...
Create Network
Aware Widgets #
Type 1: A common widget for the entire app #
STEP 1: Wrap MaterialApp/CupertinoApp
with ConnectivityAppWrapper
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return ConnectivityAppWrapper(
app: MaterialApp(
title: 'Connectivity Wrapper Example',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MenuScreen(),
builder: (buildContext, widget) {
return ConnectivityWidgetWrapper(
child: widget,
disableInteraction: true,
height: 80,
);
},
),
);
}
}
Type 2: Screen/widget specific widgets #
STEP 1: Wrap MaterialApp/CupertinoApp
with ConnectivityAppWrapper
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return ConnectivityAppWrapper(
app: MaterialApp(
title: 'Connectivity Wrapper Example',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MenuScreen(),
),
);
}
}
STEP 2: The last step, Wrap your body widget with ConnectivityWidgetWrapper
or use ConnectivityScreenWrapper
for In-build animation
class MenuScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Connectivity Wrapper Example"),
),
body: ConnectivityWidgetWrapper( // or use ##ConnectivityScreenWrapper for In build animation
child: ListView(
children: <Widget>[
ListTile(
title: Text(Strings.example1),
onTap: () {
AppRoutes.push(context, ScaffoldExampleScreen());
},
),
Divider(),
ListTile(
title: Text(Strings.example2),
onTap: () {
AppRoutes.push(context, CustomOfflineWidgetScreen());
},
),
Divider(),
],
),
),
);
}
}
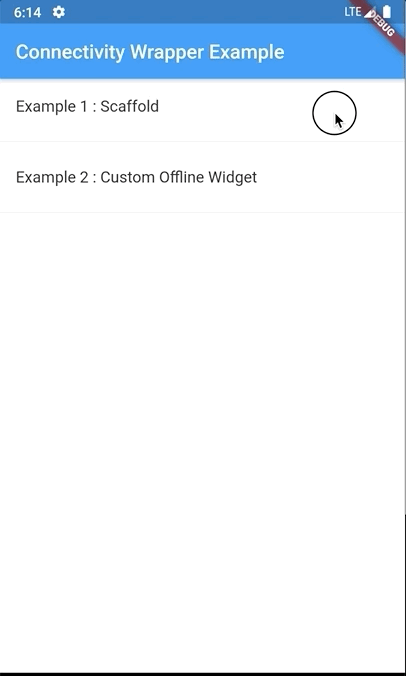
Also, you can customize the offlineWidget . Let's see few examples.
Custom Decoration #
....
body: ConnectivityWidgetWrapper(
decoration: BoxDecoration(
color: Colors.purple,
gradient: new LinearGradient(
colors: [Colors.red, Colors.cyan],
),
),
child: ListView(
....
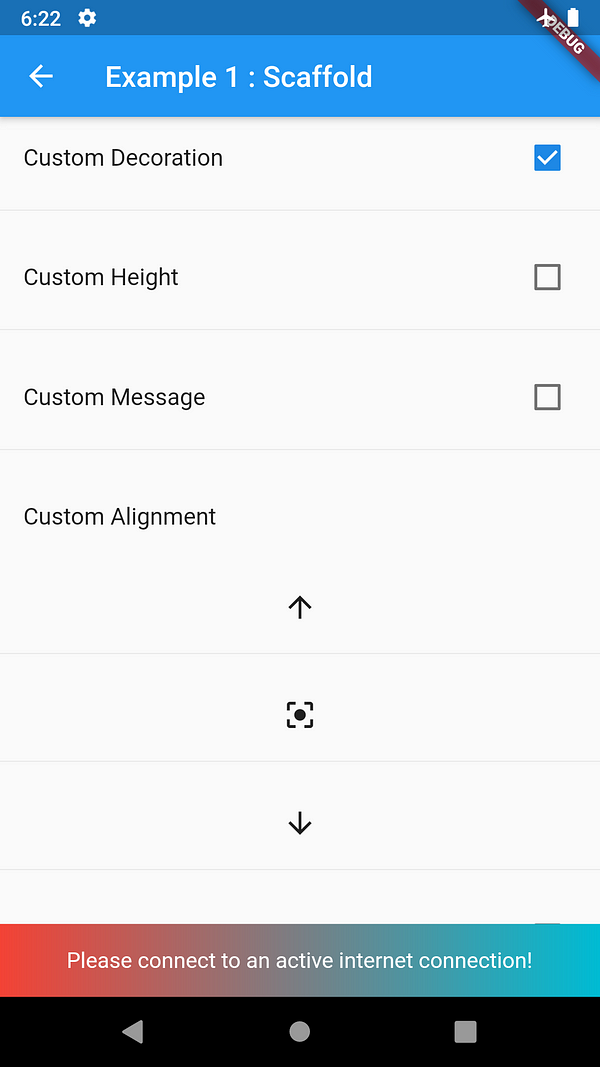
Custom Height and Message #
...
body: ConnectivityWidgetWrapper(
decoration: BoxDecoration(
color: Colors.purple,
gradient: new LinearGradient(
colors: [Colors.red, Colors.cyan],
),
),
height: 150.0,
message: "You are Offline!",
messageStyle: TextStyle(
color: Colors.white,
fontSize: 40.0,
),
child: ListView(
...
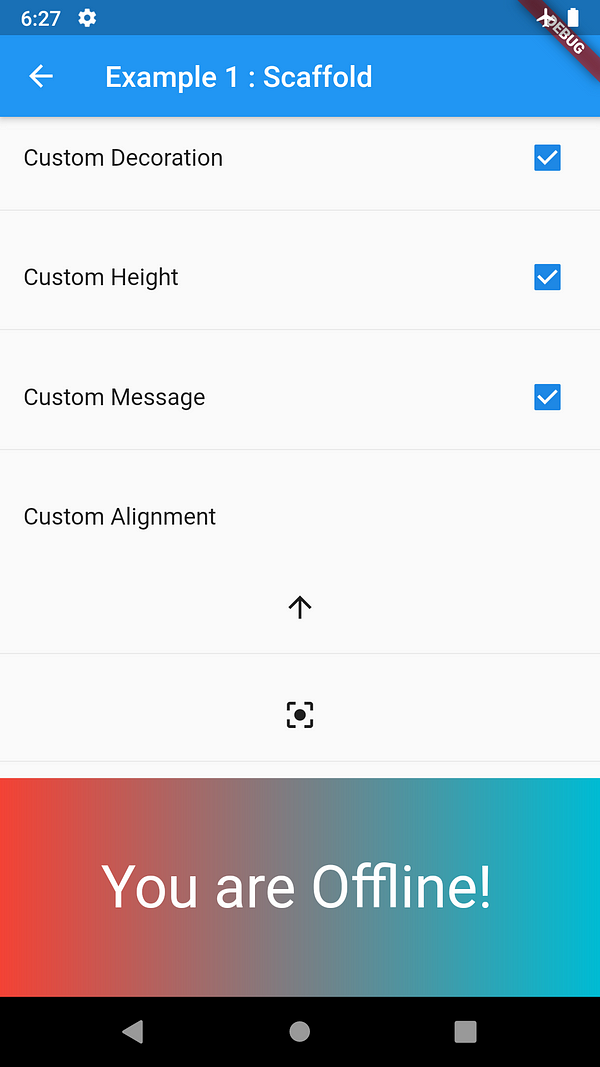
Custom Alignment and Disable User Interaction #
...
body: ConnectivityWidgetWrapper(
alignment: Alignment.topCenter,
disableInteraction: true,
child: ListView(
...
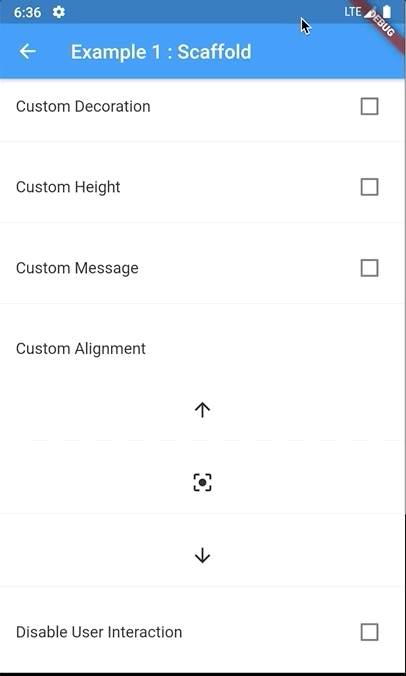
Provide your own Custom Offline Widget #
...
body: ConnectivityWidgetWrapper(
disableInteraction: true,
offlineWidget: OfflineWidget(),
child: ListView.builder(
....
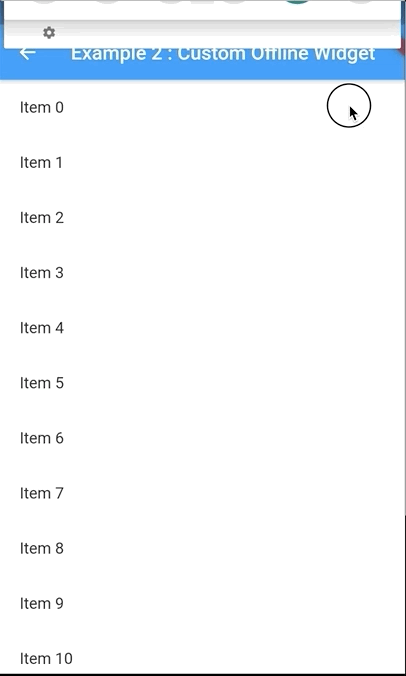
Convert Any widget to network-aware widget #
Wrap the widget RaisedButton
which you want to be network-aware with ConnectivityWidgetWrapper
and set stacked: false
.
Provide an offlineWidget
to replace the current widget when the device is offline.
class NetworkAwareWidgetScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(Strings.example3),
),
body: ListView(
padding: EdgeInsets.all(20.0),
children: <Widget>[
TextField(
decoration: InputDecoration(labelText: 'Email'),
),
P5(),
TextField(
decoration: InputDecoration(labelText: 'Password'),
),
P5(),
ConnectivityWidgetWrapper(
stacked: false,
offlineWidget: RaisedButton(
onPressed: null,
color: Colors.grey,
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
"Connecting",
style: TextStyle(
color: Colors.white,
),
),
P5(),
CupertinoActivityIndicator(radius: 8.0),
],
),
),
),
child: RaisedButton(
onPressed: () {},
child: Text(
"Sign In",
style: TextStyle(
color: Colors.white,
),
),
color: Colors.blue,
),
),
],
),
);
}
}
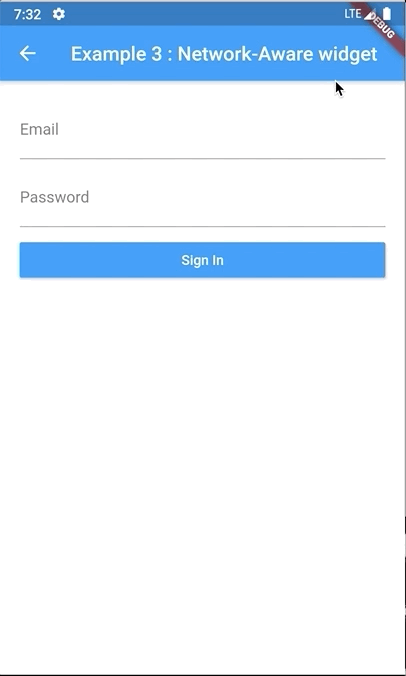
Note that you should not be using the current network status for deciding whether you can reliably make a network connection. Always guard your app code against timeouts and errors that might come from the network layer.
Contributing #
We welcome contributions in various forms:
- Proposing new features or enhancements.
- Reporting and fixing bugs.
- Engaging in discussions to help make decisions.
- Improving documentation, as it is essential.
- Sending Pull Requests is greatly appreciated!
A big thank you to all our contributors! 🙌