bottom_dropdown 0.0.4
bottom_dropdown: ^0.0.4 copied to clipboard
A new Flutter project.
bottom_dropdown #
A Flutter Package which will open DropDown below your widget.
Screenshots #
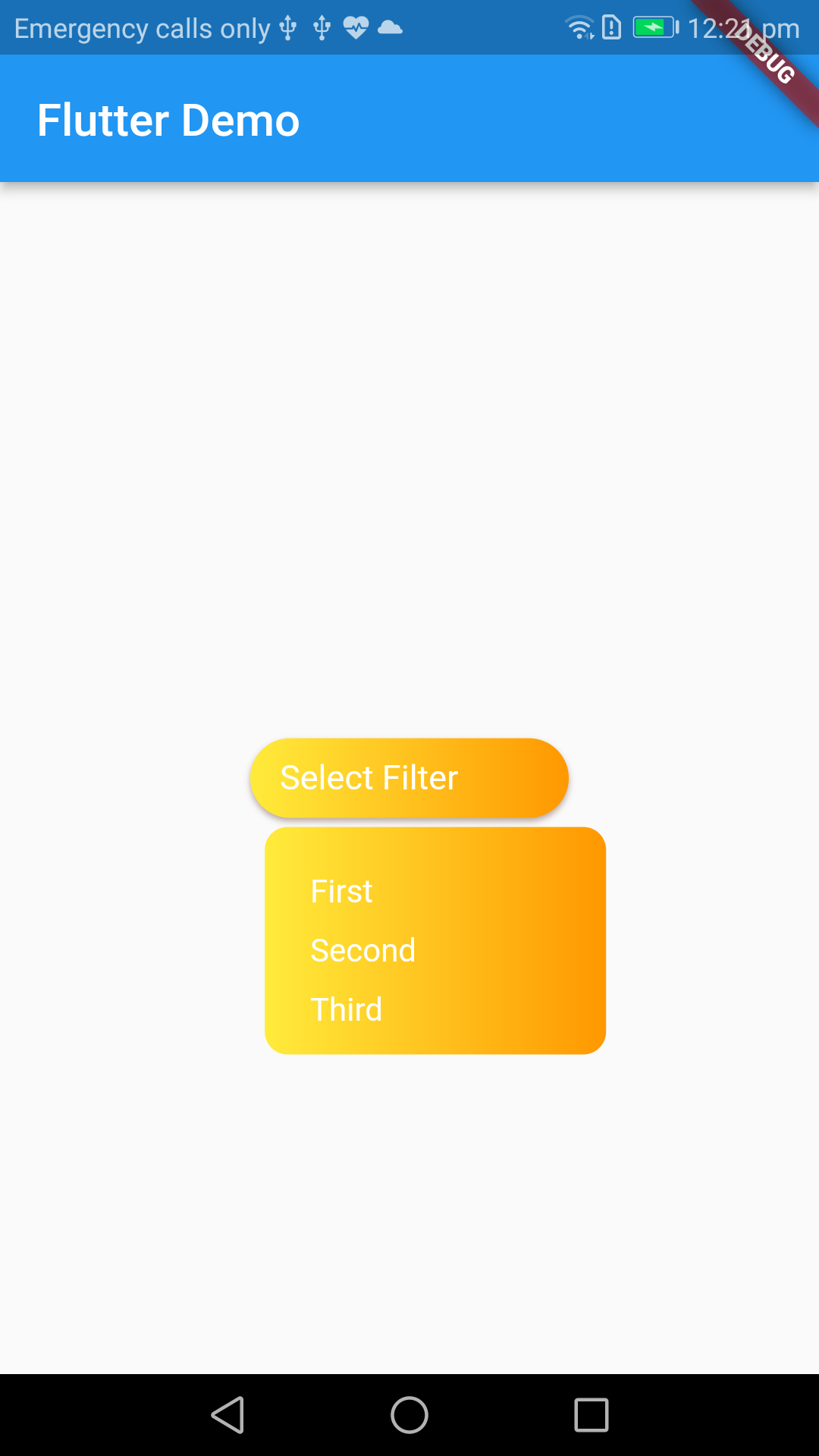
Usage #
To use this package:
- add the dependency to your pubspec.yaml file.
dependencies:
flutter:
sdk: flutter
bottom_dropdown: ^0.0.4
How to use #
import 'package:bottom_dropdown/bottom_dropdown.dart';
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Flutter Demo'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key? key, required this.title}) : super(key: key);
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
final showDropDownKey = GlobalKey();
List<KeyValueModel> myList = [
KeyValueModel(itemName: "First", itemId: "1"),
KeyValueModel(itemName: "Second", itemId: "2"),
KeyValueModel(itemName: "Third", itemId: "3"),
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: InkWell(
onTap: (){
showDropDown(
globalKey: showDropDownKey,
childHeight: 100,
context: context,
keyValueList: myList,
rightSpace: MediaQuery.of(context).size.width*0.26,
borderRadius: 10,
textAlign: TextAlign.left,
gradientBackGround: const LinearGradient(
colors: <Color>[
Colors.yellow,
Colors.orange
],
),
selectedValue: (val){
print(val.itemName);
print(val.itemId);
},
childWidth: 150,
textStyle: const TextStyle(
color: Colors.white,
)
);
},
child: Container(
key: showDropDownKey,
height: 35,
width: 140,
decoration:BoxDecoration(
borderRadius: const BorderRadius.only(
topLeft: Radius.circular(25),
bottomLeft: Radius.circular(25),
bottomRight: Radius.circular(25),
topRight: Radius.circular(25)
),
gradient: const LinearGradient(
colors: <Color>[
Colors.yellow,
Colors.orange
],
),
boxShadow: [
BoxShadow(
offset: const Offset(0.0, 1.5),
blurRadius: 1.5,
color: Colors.brown
.withOpacity(0.5)),
]
),
child: Align(
alignment: Alignment.centerLeft,
child: Padding(
padding: const EdgeInsets.only(
left: 10),
child: Row(
children: [
Container(),
const SizedBox(
width: 3,
),
SizedBox(
width:
MediaQuery.of(context)
.size
.width *
0.28,
child: const Text(
"Select Filter",
style: TextStyle(
fontSize: 15,
color: Colors.white,
fontWeight:
FontWeight
.w400),
overflow: TextOverflow
.ellipsis,
),
),
],
),
)),
),
),
),
);
}
}