beamer 0.13.3
beamer: ^0.13.3 copied to clipboard
A routing package that lets you navigate through guarded page stacks and URLs using the Router and Navigator's Pages API, aka "Navigator 2.0".
Handle your application routing, synchronize it with browser URL and more. Beamer uses the power of Router and implements all the underlying logic for you.
Quick Start #
The simplest setup is achieved by using the SimpleLocationBuilder
which yields the least amount of code for a functioning application:
class MyApp extends StatelessWidget {
final routerDelegate = BeamerDelegate(
locationBuilder: SimpleLocationBuilder(
routes: {
// Return either Widgets or BeamPages if more customization is needed
'/': (context) => HomeScreen(),
'/books': (context) => BooksScreen(),
'/books/:bookId': (context) {
// Extract the current BeamState which holds route information
final beamState = context.currentBeamLocation.state;
// Take the parameter of interest
final bookId = beamState.pathParameters['bookId']!;
// Return a Widget or wrap it in a BeamPage for more flexibility
return BeamPage(
key: ValueKey('book-$bookId'),
title: 'A Book #$bookId',
popToNamed: '/',
type: BeamPageType.scaleTransition,
child: BookDetailsScreen(bookId),
);
}
},
),
);
@override
Widget build(BuildContext context) {
return MaterialApp.router(
routeInformationParser: BeamerParser(),
routerDelegate: routerDelegate,
);
}
}
Navigating through those routes can be done with
Beamer.of(context).beamToNamed('/books/2');
Accessing route attributes (for example, bookId
for building BookDetailsScreen
) can be done with
Beamer.of(context).currentBeamLocation.state.pathParameters['bookId'];
Passing additional arbitrary attributes that don't contribute to URI can be done via data
;
Beamer.of(context).beamToNamed(
'/book/2',
data: {
'note': 'this is my favorite book',
'color': Colors.blue,
},
);
For those who wish to have a full control over building a page stack, we now introduce some key concepts; BeamLocation
and BeamState
.
Key Concepts #
At the highest level, Beamer
is a wrapper for Router
and uses its own implementations for RouterDelegate
and RouteInformationParser
. The goal of beamer is to separate the responsibility of building a page stack for Navigator.pages
into multiple classes with custom "states", instead of one global state.
For example, we would like to handle all the profile related page stacks such as
[ ProfilePage ]
,[ ProfilePage, FriendsPage]
,[ ProfilePage, FriendsPage, FriendPage ]
,[ ProfilePage, SettingsPage ]
,- ...
with some "ProfileHandler" that knows which "state" corresponds to which page stack and updates this state as the page stack changes. Then similarly, we would like to have a "ShopHandler" for all the possible stacks of shop related pages such as
[ ShopPage ]
,[ ShopPage, CategoriesPage ]
,[ ShopPage, CategoriesPage, ItemsPage ]
,[ ShopPage, CategoriesPage, ItemsPage, ItemPage ]
,[ ShopPage, ItemsPage, ItemPage ]
,[ ShopPage, CartPage ]
,- ...
These "Handlers" are called BeamLocation
s.
BeamLocation
s cannot work by themselves. When the URI
comes into the app through deep-link, or as initial, there must be a decision which BeamLocation
will further handle this URI
and build pages for the Navigator
. This is the job of BeamerDelegate.locationBuilder
that will take the "global state" and give it to appropriate BeamLocation
which will create and save its own "local state" from it to use it to build pages.
BeamLocation #
The most important construct in Beamer is a BeamLocation
which represents a stack of one or more pages.
BeamLocation
has 3 important roles:
- know which URIs it can handle:
pathBlueprints
- know how to build a stack of pages:
buildPages
- keep a
state
that provides a link between the first 2
BeamLocation
is an abstract class which needs to be extended. The purpose of having multiple BeamLocation
s is to architecturally separate unrelated "places" in an application.
For example, BooksLocation
can handle all the pages related to books and ArticlesLocation
everything related to articles. In the light of this scoping, BeamLocation
also has a builder
for wrapping an entire stack of its pages with some Provider
so the similar data can be shared between similar pages.
This is an example of BeamLocation
:
class BooksLocation extends BeamLocation {
BooksLocation(BeamState state) : super(state);
@override
List<String> get pathBlueprints => ['/books/:bookId'];
@override
List<BeamPage> buildPages(BuildContext context, BeamState state) => [
BeamPage(
key: ValueKey('home'),
child: HomeScreen(),
),
if (state.uri.pathSegments.contains('books'))
BeamPage(
key: ValueKey('books'),
child: BooksScreen(),
),
if (state.pathParameters.containsKey('bookId'))
BeamPage(
key: ValueKey('book-${state.pathParameters['bookId']}'),
child: BookDetailsScreen(
bookId: state.pathParameters['bookId'],
),
),
];
}
BeamState #
This is the above-mentioned state
of BeamLocation
. Its role is to keep various URI attributes such as pathBlueprintSegments
(the segments of chosen pathBlueprint, as each BeamLocation
supports many of those), pathParameters
, queryParameters
and arbitrary key-value data
. Those attributes are important while building pages and for BeamState
to create an uri
that will be consumed by the browser.
Besides purely imperative navigation via e.g. beamToNamed('/books/3')
, this also provides a method to have declarative navigation by changing the state
of BeamLocation
. For example:
Beamer.of(context).currentBeamLocation.update(
(state) => state.copyWith(
pathBlueprintSegments: ['books', ':bookId'],
pathParameters: {'bookId': '3'},
),
),
BeamState
can be extended with a completely custom state which can be used for BeamLocation
, for example:
class BooksLocation extends BeamLocation<MyState> {...}
It is important in this case that MyState
has an uri
getter which is needed for browser's URL bar.
Beaming #
Navigating between or within BeamLocation
s is achieved by "beaming". You can think of it as teleporting (beaming) to another place in your app. Similar to Navigator.of(context).pushReplacementNamed('/my-route')
, but Beamer is not limited to a single page, nor to a push per se. BeamLocation
s hold an arbitrary stack of pages that get built when you beam there. Using Beamer can feel like using many of Navigator
's push/pop
methods at once.
Examples of beaming:
Beamer.of(context).beamTo(MyLocation());
// or with an extension on BuildContext
context.beamTo(MyLocation());
context.beamToNamed('/books/2');
// this is equivalent to
context.beamTo(
BooksLocation(
BeamState(
pathBlueprintSegments: ['books', ':bookId'],
pathParameters: {'bookId': '2'},
),
),
),
context.beamToNamed(
'/book/2',
data: {'note': 'this is my favorite book'},
);
Updating #
Once at a BeamLocation
, it is preferable to update the current location's state. For example, for going from /books
to /books/3
(which are both handled by BooksLocation
):
context.currentBeamLocation.update(
(state) => state.copyWith(
pathBlueprintSegments: ['books', ':bookId'],
pathParameters: {'bookId': '3'},
),
),
To get from one BeamLocation
's stack to another BeamLocation
's stack, an update
can be invoked on BeamerDelegate
itself:
Beamer.of(context).update(
state: BeamState(
pathBlueprintSegments: ['articles', ':articleId'],
pathParameters: {'articleId': '1'},
),
);
NOTE that every beaming function (beamTo
, beamToNamed
,...) will have the same effect as update
either on BeamLocation
or on BeamerDelegate
.
Beaming Back #
NOTE: Navigating to previous page in a page stack is done via Navigator.of(context).pop()
. This is also what the default AppBar
's BackButton
will call. If you beamed to the current page stack from some different page stack, then consider beamBack
to return to your previous configuration.
All BeamState
s that were visited are kept in beamStateHistory
. Therefore, there is an ability to beam back to whichever BeamLocation
is responsible for previous BeamState
. For example, after spending some time on /books
and /books/3
, say you beam to /articles
. From there, you can get back to your previous location as it were when you left, i.e. /books/3
.
context.beamBack();
NOTE that Beamer can integrate Android's back button to do beamBack
if possible when all the pages from current BeamLocation
have been popped. This is achieved by setting a back button dispatcher in MaterialApp.router
.
backButtonDispatcher: BeamerBackButtonDispatcher(delegate: routerDelegate)
Usage #
To use the full-featured Beamer in your app, you must (as per official documentation) construct your *App
widget with .router
constructor to which (along with all your regular *App
attributes) you provide
routeInformationParser
that parses an incoming URI.routerDelegate
that controls (re)building ofNavigator
Here you use the Beamer implementation of those - BeamerParser
and BeamerDelegate
, to which you pass your LocationBuilder
.
In the simplest form, LocationBuilder
is just a function which takes the current BeamState
and returns a custom BeamLocation
based on the URI or other state properties.
class MyApp extends StatelessWidget {
final routerDelegate = BeamerDelegate(
locationBuilder: (state) {
if (state.uri.pathSegments.contains('books')) {
return BooksLocation(state);
}
return HomeLocation(state);
},
);
@override
Widget build(BuildContext context) {
return MaterialApp.router(
routerDelegate: routerDelegate,
routeInformationParser: BeamerParser(),
backButtonDispatcher:
BeamerBackButtonDispatcher(delegate: routerDelegate),
);
}
}
There are also two other options available, if you don't want to define a custom LocationBuilder
function.
With a List of BeamLocations #
You can use the BeamerLocationBuilder
with a list of BeamLocation
s. This builder will automatically select the correct location, based on the pathBlueprints
of each BeamLocation
. In this case, define your BeamerDelegate
like this:
final routerDelegate = BeamerDelegate(
locationBuilder: BeamerLocationBuilder(
beamLocations: [
HomeLocation(),
BooksLocation(),
],
),
);
With a Map of Routes #
You can use the SimpleLocationBuilder
with a map of routes and WidgetBuilder
s, as mentioned in Quick Start. This completely removes the need for custom BeamLocation
s, but also gives you the least amount of customizability. Still, wildcards and path parameters in your paths are supported as with all the other options.
final routerDelegate = BeamerDelegate(
locationBuilder: SimpleLocationBuilder(
routes: {
'/': (context) => HomeScreen(),
'/books': (context) => BooksScreen(),
'/books/:bookId': (context) => BookDetailsScreen(
bookId: context.currentBeamLocation.state.pathParameters['bookId'],
),
},
),
);
Nested Navigation #
When nested navigation is needed, you can just put Beamer
anywhere in the Widget tree where this navigation will take place. There is no limit on how many Beamer
s an app can have. Common use case is a bottom navigation bar (see example), something like this:
class MyApp extends StatelessWidget {
final routerDelegate = BeamerDelegate(
initialPath: '/books',
locationBuilder: SimpleLocationBuilder(
routes: {
'/*': (context) {
final beamerKey = GlobalKey<BeamerState>();
return Scaffold(
body: Beamer(
key: beamerKey,
routerDelegate: BeamerDelegate(
locationBuilder: BeamerLocationBuilder(
beamLocations: [
BooksLocation(),
ArticlesLocation(),
],
),
),
),
bottomNavigationBar: BottomNavigationBarWidget(
beamerKey: beamerKey,
),
);
}
},
),
);
@override
Widget build(BuildContext context) {
return MaterialApp.router(
routerDelegate: routerDelegate,
routeInformationParser: BeamerParser(),
);
}
}
General Notes #
-
When extending
BeamLocation
, two methods need to be implemented:pathBlueprints
andbuildPages
.buildPages
returns a stack of pages that will be built byNavigator
when you beam there, andpathBlueprints
is there for Beamer to decide whichBeamLocation
corresponds to which URI.BeamLocation
keeps query and path parameters from URI in itsBeamState
. The:
is necessary inpathBlueprints
if you might get path parameter from browser.
-
BeamPage
's child is an arbitraryWidget
that represents your app screen / page.key
is important forNavigator
to optimize rebuilds. This should be a unique value for "page state".BeamPage
createsMaterialPageRoute
by default, but other transitions can be chosen by settingBeamPage.type
to one of availableBeamPageType
.
NOTE that "Navigator 1.0" can be used alongside Beamer. You can easily push
or pop
pages with Navigator.of(context)
, but those will not be contributing to the URI. This is often needed when some info/helper page needs to be shown that doesn't influence the browser's URL. And of course, when using Beamer on mobile, this is a non-issue as there is no URL.
Tips and Common Issues #
- removing the
#
from URL can be done by callingBeamer.setPathUrlStrategy()
beforerunApp()
. BeamPage.title
is used for setting the browser tab title by default and can be opt-out by settingBeamerDelegate.setBrowserTabTitle
tofalse
.- Losing state on hot reload
Examples #
Check out all examples here.
Location Builders #
Here is a recreation of the example app from this article where you can learn a lot about Navigator 2.0. It contains three different options of building the locations. The full code is available here.
Advanced Books #
For a step further, we add more flows to demonstrate the power of Beamer. The full code is available here.
Deep Location #
You can instantly beam to a location in your app that has many pages stacked (deep linking) and then pop them one by one or simply beamBack
to where you came from. The full code is available here. Note that beamBackOnPop
parameter of beamToNamed
might be useful here to override AppBar
's pop
with beamBack
.
ElevatedButton(
onPressed: () => context.beamToNamed('/a/b/c/d'),
//onPressed: () => context.beamToNamed('/a/b/c/d', beamBackOnPop: true),
child: Text('Beam deep'),
),
Provider #
You can override BeamLocation.builder
to provide some data to the entire location, i.e. to all the pages
. The full code is available here.
// In your location implementation
@override
Widget builder(BuildContext context, Navigator navigator) {
return MyProvider<MyObject>(
create: (context) => MyObject(),
child: navigator,
);
}
Guards #
You can define global guards (for example, authentication guard) or location guards that keep a specific location safe. The full code is available here.
- Global Guards
BeamerDelegate(
guards: [
// Guard /books and /books/* by beaming to /login if the user is unauthenticated:
BeamGuard(
pathBlueprints: ['/books', '/books/*'],
check: (context, location) => context.isAuthenticated,
beamToNamed: '/login',
),
],
...
),
- Location (local) Guards
// in your location implementation
@override
List<BeamGuard> get guards => [
// Show forbiddenPage if the user tries to enter books/2:
BeamGuard(
pathBlueprints: ['/books/2'],
check: (context, location) => false,
showPage: forbiddenPage,
),
];
Authentication Bloc #
Here is an example on how to use BeamGuard
s for an authentication flow. It uses flutter_bloc for state management. The code is available here.
Bottom Navigation #
An examples of putting Beamer
into the Widget tree is when using a bottom navigation bar. The code is available here.
Bottom Navigation Multiple Beamers #
The code for the bottom navigation example app with multiple beamers is available here
Nested Navigation #
NOTE: In all nested Beamer
s, full paths must be specified when defining BeamLocation
s and beaming. (support for relative paths is in progress)
The code for the nested navigation example app is available here
Integration with Navigation UI Packages #
- Animated Rail Example, with animated_rail package.
- ... (Contributions are very welcome! Add your suggestion here or make a PR.)
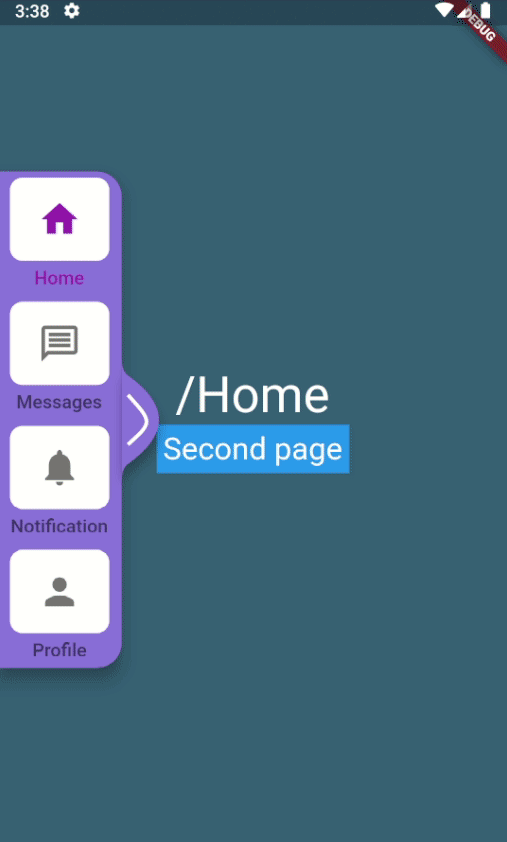
Migrating #
From 0.12 to 0.13 #
- rename
BeamerRouterDelegate
toBeamerDelegate
- rename
BeamerRouteInformationParser
toBeamerParser
- rename
pagesBuilder
tobuildPages
- rename
Beamer.of(context).currentLocation
toBeamer.of(context).currentBeamLocation
From 0.11 to 0.12 #
- There's no
RootRouterDelegate
anymore. Just rename it toBeamerDelegate
. If you were using itshomeBuilder
, useSimpleLocationBuilder
and thenroutes: {'/': (context) => HomeScreen()}
. - Behavior of
beamBack
was changed to go to previousBeamState
, notBeamLocation
. If this is not what you want, usepopBeamLocation()
that has the same behavior as oldbeamback
.
From 0.10 to 0.11 #
BeamerDelegate.beamLocations
is nowlocationBuilder
. SeeBeamerLocationBuilder
for easiest migration.Beamer
now takesBeamerDelegate
, notBeamLocations
directlybuildPages
now also bringsstate
From 0.9 to 0.10 #
BeamLocation
constructor now takes onlyBeamState state
. (there's no need to define special constructors and callsuper
if you usebeamToNamed
)- most of the attributes that were in
BeamLocation
are now inBeamLocation.state
. When accessing them throughBeamLocation
:pathParameters
is nowstate.pathParameters
queryParameters
is nowstate.queryParameters
data
is nowstate.data
pathSegments
is nowstate.pathBlueprintSegments
uri
is nowstate.uri
From 0.7 to 0.8 #
- rename
pages
tobuildPages
inBeamLocation
s - pass
beamLocations
toBeamerDelegate
instead ofBeamerParser
. See Usage
From 0.4 to 0.5 #
- instead of wrapping
MaterialApp
withBeamer
, use*App.router()
String BeamLocation.pathBlueprint
is nowList<String> BeamLocation.pathBlueprints
BeamLocation.withParameters
constructor is removed and all parameters are handled with 1 constructor. See example if you needsuper
.BeamPage.page
is now calledBeamPage.child
Help and Chat #
For any problems, questions, suggestions, fun,... join us at Discord
Contributing #
This package is still in early stages. To see the upcoming features, check the Issue board.
If you notice any bugs not present in issues, please file a new issue. If you are willing to fix or enhance things yourself, you are very welcome to make a pull request. Before making a pull request:
- if you wish to solve an existing issue, please let us know in issue comments first.
- if you have another enhancement in mind, create an issue for it first, so we can discuss your idea.