android_dynamic_icon 2.0.0
android_dynamic_icon: ^2.0.0 copied to clipboard
A flutter plugin for dynamically changing multiple android app icons for your app.
android_dynamic_icon #
A flutter plugin for dynamically changing multiple android app icons for your app. Supports only Android.
Usage #
To use this plugin, add android_dynamic_icon
as a dependency in your pubspec.yaml file.
Getting Started #
Check out the example
directory for a sample app using android_dynamic_icon
Integration #
-
Add the latest version of the plugin to your
pubpsec.yaml
under dependencies section -
Run
flutter pub get
-
Add your all icons on
android/src/main/res/drawable
folder -
For having multiple app icon in your app you should note following:
- For each icon, you must declare an activity-alias in
AndroidManifest.xml
(see example in point 5) - For each icon you add, make a
ExampleIcon.kt
file belowMainActivity.kt
file (see example in point 6)
- For each icon, you must declare an activity-alias in
-
Update
android/src/main/AndroidManifest.xml
as follows:<application...> <activity android:name=".MainActivity" android:exported="true" android:launchMode="singleTop" android:theme="@style/LaunchTheme" android:configChanges="orientation|keyboardHidden|keyboard|screenSize|smallestScreenSize|locale|layoutDirection|fontScale|screenLayout|density|uiMode" android:hardwareAccelerated="true" android:windowSoftInputMode="adjustResize"> <meta-data android:name="io.flutter.embedding.android.NormalTheme" android:resource="@style/NormalTheme" /> <action android:name="android.intent.action.MAIN"/> <category android:name="android.intent.category.LAUNCHER"/> </intent-filter> </activity> <!--name activity alias for icons you want to use--> <activity-alias android:label="app" android:icon="@drawable/iconone" android:name=".IconOne" android:enabled="false" android:exported="true" android:targetActivity=".MainActivity"> <!--target activity class path will be same for all alias--> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity-alias> <activity-alias android:label="app" android:icon="@drawable/icontwo" android:name=".IconTwo" android:enabled="false" android:exported="true" android:targetActivity=".MainActivity"> <!--target activity class path will be same for all alias--> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity-alias> </application>
-
Add
IconOne.kt
andIconTwo.kt
belowMainActivity.kt
as follows:package com.example.android_dynamic_icon import io.flutter.embedding.android.FlutterActivity class IconOne: FlutterActivity() { }
package com.example.android_dynamic_icon import io.flutter.embedding.android.FlutterActivity class IconTwo: FlutterActivity() { }
Dart/Flutter Integration #
From your Dart code, you need to import the plugin and use it's methods:
- First Initiliaze the plugin by all classes you made Including MainActivity as it is responsible for Default icon.
import 'package:android_dynamic_icon/android_dynamic_icon.dart';
final _androidDynamicIconPlugin = AndroidDynamicIcon();
@override
void initState() {
AndroidDynamicIcon.initialize(
classNames: ['MainActivity', 'IconOne', 'IconTwo']);
super.initState();
}
- Now set different icons using change icon method.
//To set Icon One
await _androidDynamicIconPlugin
.changeIcon(classNames: ['IconOne', '']);
//To set Icon Two
await _androidDynamicIconPlugin
.changeIcon(classNames: ['IconOne', '']);
//To set Default Icon
await _androidDynamicIconPlugin
.changeIcon(classNames: ['MainActivity', '']);
Check out the example
app for more details
Demo #
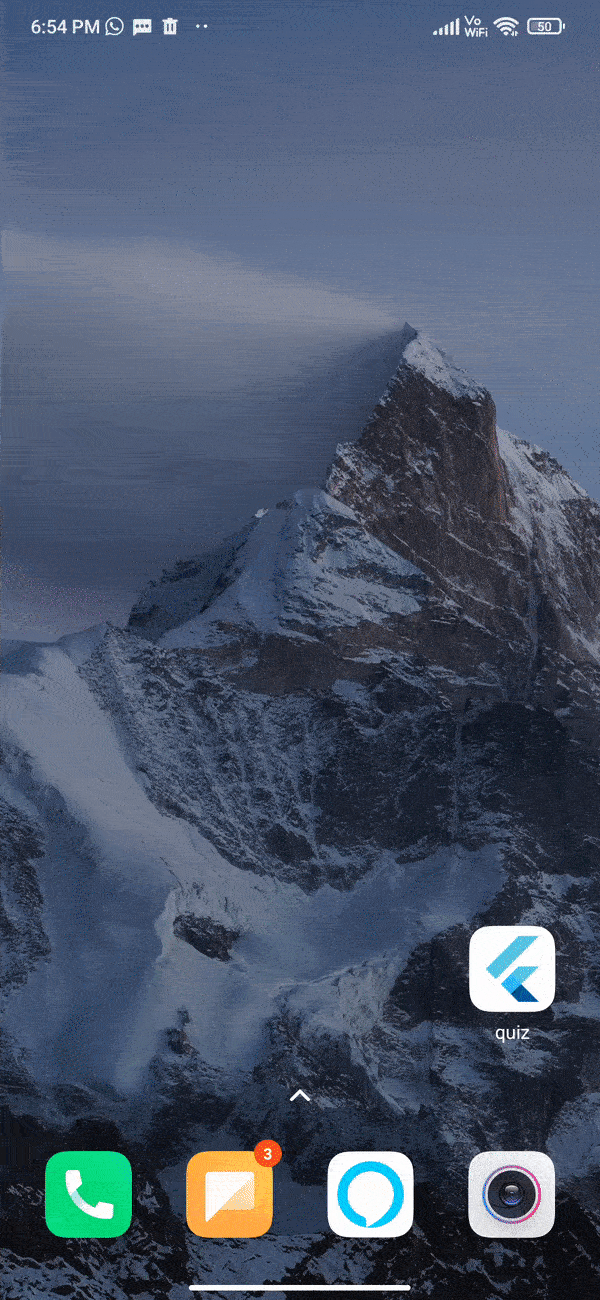