Design Credit & screen recording
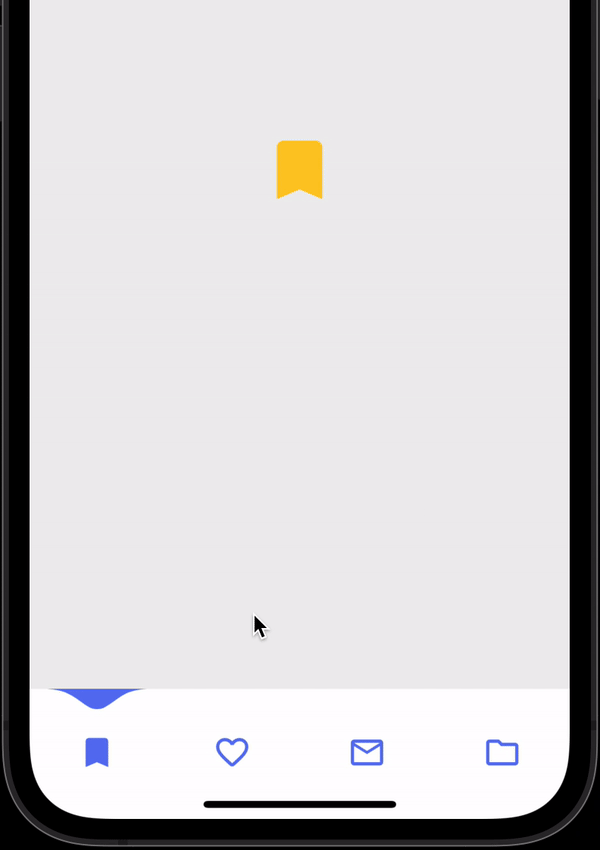
How to use?
Installation
Add water_drop_nav_bar:
to your pubspec.yaml
dependencies then run flutter pub get
dependencies:
water_drop_nav_bar:
Import
Add this line to import the package.
import 'package:water_drop_nav_bar/water_drop_nav_bar.dart';
Add WaterDropNavBar()
as bottomNavigationBar
of Scaffold()
and body would be PageView()
with NeverScrollableScrollPhysics()
don't try to upate the seleted index from onPageChanged
or will see some weird behaviour. Insted of PageView()
You can use Stack()
or AnimatedSwitcher for custom page transition animation.
API Reference
barItems → List<BarItem>
- List of bar items that shows horizontally, Minimum 2 and maximum 4 items.
required
onItemSelected → OnButtonPressCallback
- Callback When individual barItem is pressed.
required
selectedIndex → int
- Current selected index of the bar item.
required
backgroundColor → Color
- Background Color of the bar.
optional Colors.white
waterDropColor → Color
- Color of water drop which is also the active icon color.
optional Color(0xFF5B75F0)
inactiveIconColor → Color
- Inactive icon color by default it will use water drop color.
optionalwaterDropColor
iconSize → double
- Each active & inactive icon size, default value is 28 don't make it too big or small.
optional28
bottomPadding → double
- Additional padding at the bottom of the bar. If nothing is provided the it will use
MediaQuery.of(context).padding.bottom value.
optional
Do and don't
- Don't make icon size too big.
- Use complementary filled and outlined icons for best result.
backgroundColor
andwaterDropColor
ofWaterDropNavBar()
andScaffold()
'sbackgroundColor
(or whatever widget you are using) must be different (see the example app) This will visualize that the water drop is hanging from the top.
Short example
return Scaffold(
body: PageView(
physics: NeverScrollableScrollPhysics(),
controller: pageController,
...
),
bottomNavigationBar: WaterDropNavBar(
backgroundColor: Colors.white,
onItemSelected: (index) {
setState(() {
selectedIndex = index;
});
pageController.animateToPage(selectedIndex,
duration: const Duration(milliseconds: 400),
curve: Curves.easeOutQuad);
},
selectedIndex: selectedIndex,
barItems: [
BarItem(
filledIcon: Icons.bookmark_rounded,
outlinedIcon: Icons.bookmark_border_rounded,
),
BarItem(
filledIcon: Icons.favorite_rounded,
outlinedIcon: Icons.favorite_border_rounded),
],
),
);
Issues ❗️
Android
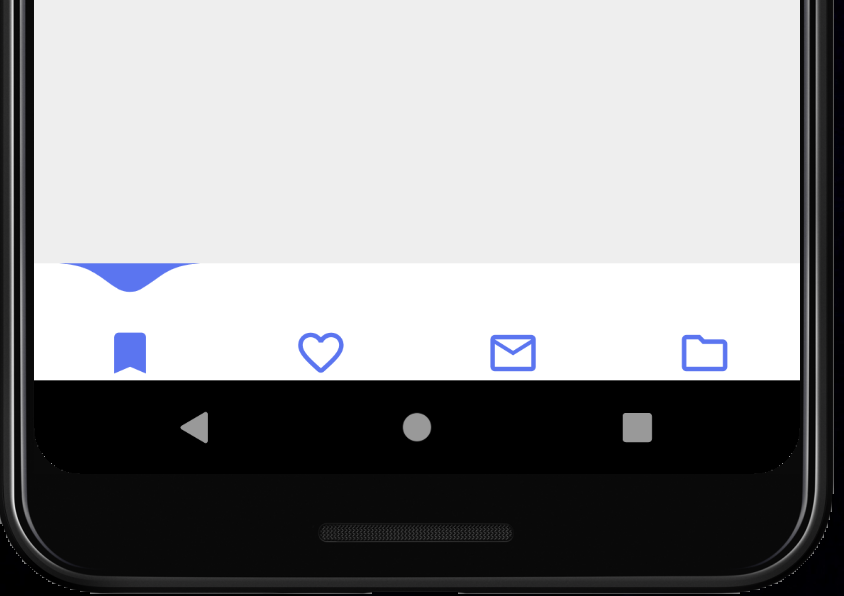
Some android phones might have black navigation bar, this looks ugly. It's recommended to wrap Scaffold
with AnnotatedRegion<SystemUiOverlayStyle>
to change that black navigation bar color to WaterDropNavBar
backgroundColor
. Check the example app. Like this 👇
return AnnotatedRegion<SystemUiOverlayStyle>(
value: const SystemUiOverlayStyle(
//this color must be equal to the WaterDropNavBar backgroundColor
systemNavigationBarColor: Colors.white,
systemNavigationBarIconBrightness: Brightness.dark,
),
child: Scaffold(
body: // code here
)
);
result 👇
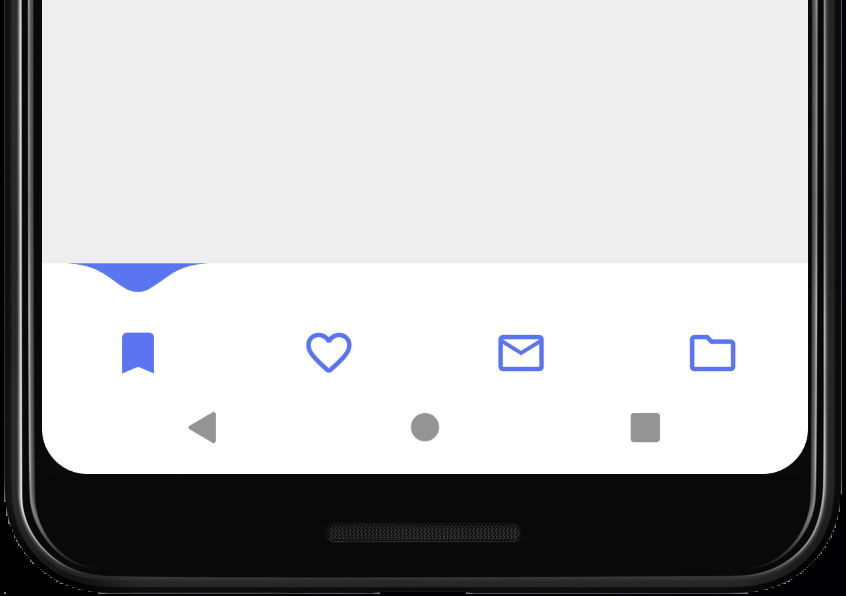
You can additionally provide some bottomPadding
to add padding at the bottom of the bar, I think 8 is enough.
iPhone
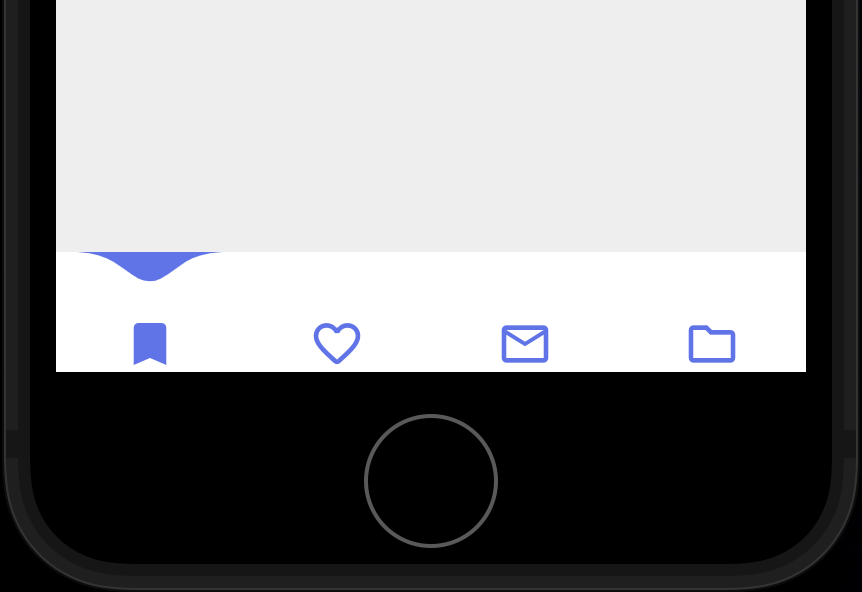
iPhones without swipe home gesture might have such issue where icons are pushed to the bottom. Provide some bottomPadding
. I added 8 padding here.
result 👇
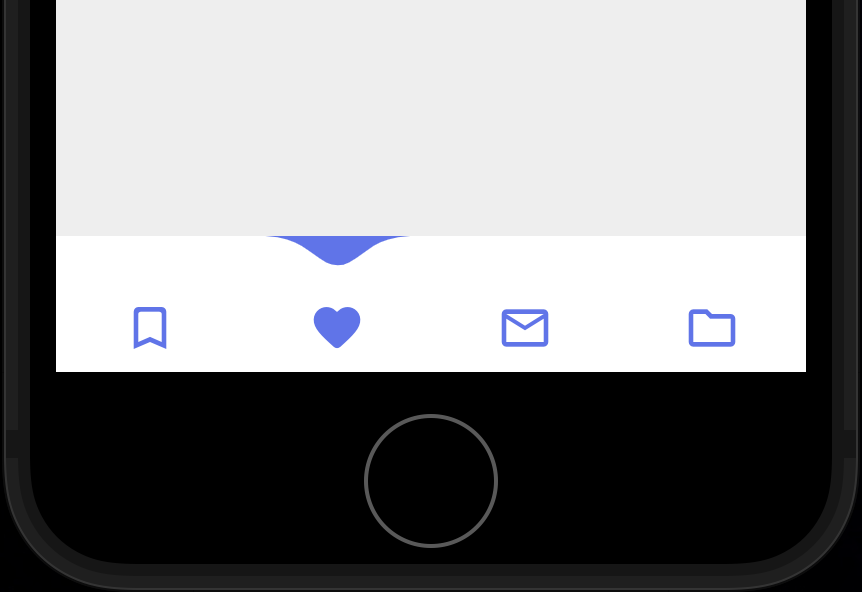
Now you might ask how do you know which phone is using swipe home gesture?
Well, you can check bottom padding (using MediaQuery.of(context).padding.bottom
) and if it's less than 34 or something then provide some bottom padding. Definitely try running different simulators and see.
FAQ
-
How do I change the height?
The height must be constant because the animation is in vertical direction. According to me 60 is perfect. But if you think needs to be reduced then please create an issue with a screenshot. I will see if I can do something.
-
How do I add drop shadow?
Wrap WaterDropNavBar
with DecoratedBox
or Container
and pass BoxDecoration
to decoration
property. BoxDecoration
takes list of boxShadow
there you can pass your drop shadow.
DecoratedBox(
decoration: BoxDecoration(
boxShadow: [
BoxShadow(
color: Colors.black.withOpacity(0.2),
offset: Offset(0, 4),
blurRadius: 8.0)
],
),
child: WaterDropNavBar()
)
-
How do I change the corner radius of the navigation bar?
Wrap WaterDropNavBar
with ClipRRect and pass BorderRadius
to borderRadius
property.
ClipRRect(
borderRadius: const BorderRadius.vertical(
top: Radius.circular(16),
),
child: WaterDropNavBar(
)
All flutter packages
● Sliding Clipped Nav Bar
➜ Water Drop Nav Bar
● Swipeable Tile
● Loading Animation Widget