⭐️Show some ❤️ and star the repo.⭐
A highly customizable, simple and easy to use searchfield widget. This Widget allows you to search and select a suggestion from list of suggestions. Think of this widget like a dropdownButton field with the following capabilities
- search 🔍 from a list of suggestions.
- validate the input with custom validation logic.
- Dynamic height of each suggestion item.
- show suggestions of custom type (not just String)
- lazy load the suggestions from Network with custom Loading widget
- show dynamic suggestions above or below the input field
- define max number of items visible in the viewport 📱
- filter out the suggestions with a custom logic
- visually customize the input and the suggestions
- navigate through the suggestions using keyboard
- Listen to scroll events of suggestions
Getting Started
Installation
- Add the dependency
flutter pub add searchfield
- Import the package
import 'package:searchfield/searchfield.dart';
Use the Widget
Example1
SearchField<Country>(
suggestions: countries
.map(
(e) => SearchFieldListItem<Country>(
e.name,
item: e,
// Use child to show Custom Widgets in the suggestions
// defaults to Text widget
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Row(
children: [
CircleAvatar(
backgroundImage: NetworkImage(e.flag),
),
SizedBox(
width: 10,
),
Text(e.name),
],
),
),
),
).toList(),
),
Example 2 (Network demo)
Ability to load suggestions from the network with a custom loading widget
SearchField(
onSearchTextChanged: (query) {
final filter = suggestions
.where((element) =>
element.toLowerCase().contains(query.toLowerCase()))
.toList();
return filter
.map((e) =>
SearchFieldListItem<String>(e, child: searchChild(e)))
.toList();
},
onTap: () async {
final result = await getSuggestions();
setState(() {
suggestions = result;
});
},
/// widget to show when suggestions are empty
emptyWidget: Container(
decoration: suggestionDecoration,
height: 200,
child: const Center(
child: CircularProgressIndicator(
color: Colors.white,
))),
hint: 'Load suggestions from network',
itemHeight: 50,
scrollbarDecoration: ScrollbarDecoration(),
suggestionStyle: const TextStyle(fontSize: 24, color: Colors.white),
searchInputDecoration: InputDecoration(...),
border: OutlineInputBorder(...)
fillColor: Colors.white,
filled: true,
contentPadding: const EdgeInsets.symmetric(
horizontal: 20,
),
),
suggestionsDecoration: suggestionDecoration,
suggestions: suggestions
.map((e) => SearchFieldListItem<String>(e, child: searchChild(e)))
.toList(),
focusNode: focus,
suggestionState: Suggestion.expand,
onSuggestionTap: (SearchFieldListItem<String> x) {
focus.unfocus();
},
),
Example 3 Custom Widgets
Example 4 Load suggestions from network with Pagination
Note that this also maintains the Scroll position when new items are added to list see: complete code here
Example 5 (Validation)
Form(
key: _formKey,
child: SearchField(
suggestions: _statesOfIndia
.map((e) => SearchFieldListItem(e))
.toList(),
suggestionState: Suggestion.expand,
textInputAction: TextInputAction.next,
hint: 'SearchField Example 2',
searchStyle: TextStyle(
fontSize: 18,
color: Colors.black.withOpacity(0.8),
),
validator: (x) {
if (!_statesOfIndia.contains(x) || x!.isEmpty) {
return 'Please Enter a valid State';
}
return null;
},
searchInputDecoration: InputDecoration(
focusedBorder: OutlineInputBorder(
borderSide: BorderSide(
color: Colors.black.withOpacity(0.8),
),
),
border: OutlineInputBorder(
borderSide: BorderSide(color: Colors.red),
),
),
maxSuggestionsInViewPort: 6,
itemHeight: 50,
onTap: (x) {},
))
SearchField(
onSearchTextChanged: (query) {
final filter = suggestions
.where((element) =>
element.toLowerCase().contains(query.toLowerCase()))
.toList();
return filter
.map((e) => SearchFieldListItem<String>(e,
child: Padding(
padding: const EdgeInsets.symmetric(vertical: 4.0),
child: Text(e,
style: TextStyle(fontSize: 24, color: Colors.red)),
)))
.toList();
},
key: const Key('searchfield'),
hint: 'Search by country name',
itemHeight: 50,
searchInputDecoration:
InputDecoration(hintStyle: TextStyle(color: Colors.red)),
suggestionsDecoration: SuggestionDecoration(
padding: const EdgeInsets.all(4),
border: Border.all(color: Colors.red),
borderRadius: BorderRadius.all(Radius.circular(10))),
suggestions: suggestions
.map((e) => SearchFieldListItem<String>(e,
child: Padding(
padding: const EdgeInsets.symmetric(vertical: 4.0),
child: Text(e,
style: TextStyle(fontSize: 24, color: Colors.red)),
)))
.toList(),
focusNode: focus,
suggestionState: Suggestion.expand,
onSuggestionTap: (SearchFieldListItem<String> x) {
focus.unfocus();
},
),
));
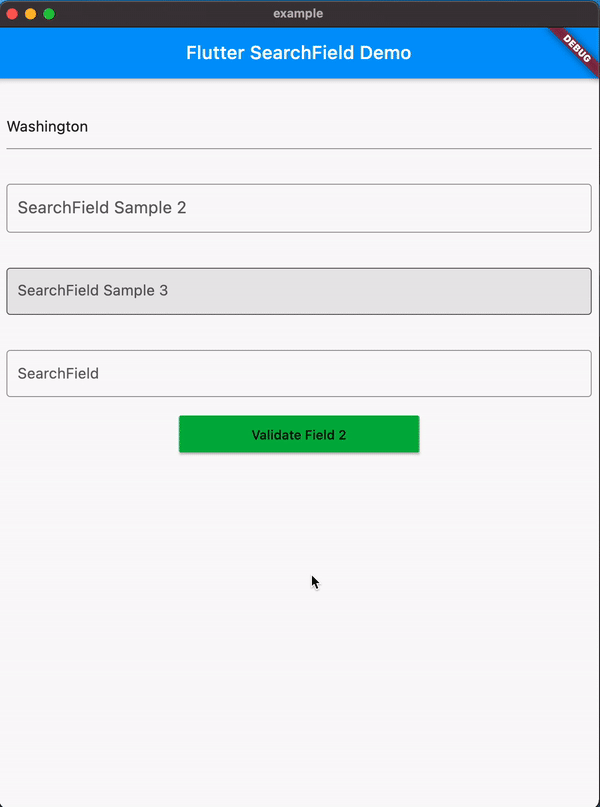
Customize the suggestions the way you want
Suggestions can be passed as a widget using the child property of SearchFieldListItem
SearchField(
suggestions: _statesOfIndia
.map((e) => SearchFieldListItem(e,
child: Align(
alignment: Alignment.centerRight,
child: Padding(
padding: const EdgeInsets.symmetric(horizontal:16.0),
child: Text(e,
style: TextStyle(color: Colors.red),
),
),
))).toList(),
...
...
)
Support for Keyboard Navigation
With v0.9.5 Searchfield now adds support for Keyboard navigation, you can now navigate through the suggestions using the keyboard.
Shortcuts:
- Up/Down Arrow keys are circular, i.e. if you reach the last item and press down, it will take you to the first item. Similarly, if you reach the first item and press up, it will take you to the last item.
ctr/option + down
to scroll to last item in the listctr/option + up
to scroll to first item in the list
Support for Dynamic positioning of suggestions
The position of suggestions is dynamic based on the space available for the suggestions to expand within the viewport.
Properties
animationDuration
: Duration for the animation of the suggestions list.autoCorrect
: Defines whether to enable autoCorrect defaults totrue
autofocus
: Defines whether to enable autofocus defaults tofalse
autoValidateMode
: Used to enable/disable this form field auto validation and update its error text.defaults toAutoValidateMode.disabled
controller
: TextEditing Controller to interact with the searchfield.comparator
property to filter out the suggestions with a custom logic (Comparator is deprecated UseonSearchTextChanged
instead).dynamicHeightItem
: Set to true to opt-in to dynamic height, defaults to false (itemHeight : 51
)emptyWidget
: Custom Widget to show when search returns empty Results or whenshowEmpty
is true. (defaults toSizedBox.shrink
)enabled
: Defines whether to enable the searchfield defaults totrue
focusNode
: FocusNode to interact with the searchfield.hint
: hint for the search Input.readOnly
: Defines whether to enable the searchfield defaults tofalse
initialValue
: The initial value to be set in searchfield when its rendered, if not specified it will be empty.inputType
: Keyboard Type for SearchFieldinputFormatters
: Input Formatter for SearchFielditemHeight
: height of each suggestion Item, (defaults to 51.0).marginColor
: Color for the margin between the suggestions.maxSuggestionBoxHeight
: Specifies a maximum height for the suggestion box whendynamicHeightItem
is set totrue
.maxSuggestionsInViewPort
: The max number of suggestions that can be shown in a viewport.offset
: suggestion List offset from the searchfield, The top left corner of the searchfield is the origin (0,0).onOutSideTap
: callback when the user taps outside the searchfield.onSaved
: An optional method to call with the final value when the form is saved via FormState.save.onScroll
: callback when the suggestion list is scrolled. It returns the current scroll position in pixels and the max scroll position.onSearchTextChanged
: callback when the searchfield text changes, it returns the current text in the searchfield.onSuggestionTap
: callback when a sugestion is tapped it also returns the tapped value.onSubmit
: callback when the searchfield is submitted, it returns the current text in the searchfield.onTap
: callback when the searchfield is tapped or brought into focus.scrollbarDecoration
: decoration for the scrollbar.showEmpty
: Boolean to show/hide the emptyWidget.suggestions
(required) : List of SearchFieldListItem to search from. eachSearchFieldListItem
in the list requires a unique searchKey, which is used to search the list and an optional Widget, Custom Object to display custom widget and to associate a object with the suggestion list.suggestionState
: enum to hide/show the suggestion on focusing the searchfield defaults toSuggestionState.expand
.searchStyle
: textStyle for the search Input.searchInputDecoration
: decoration for the search Input (e.g to update HintStyle) similar to built in textfield widget.suggestionsDecoration
: decoration for suggestions List with ability to add box shadow background color and much more.suggestionDirection
: direction of the suggestions list, defaults toSuggestionDirection.down
.suggestionItemDecoration
: decoration for suggestionItem with ability to add color and gradient in the background.SuggestionAction
: enum to control focus of the searchfield on suggestion tap.suggestionStyle
:SpecifiesTextStyle
for suggestions when no child is provided.textInputAction
: An action the user has requested the text input control to perform throgh the submit button on keyboard.textCapitalization
: Configures how the platform keyboard will select an uppercase or lowercase keyboard on IOS and Android.textAlign
: specifies the alignment of the text in the searchfield. Defaults toTextAlign.start
.
You can find all the code samples here
Contributing
You are welcome to contribute to this package, to contribute please read the contributing guidelines.
Contributors
Thanks to all the contributors who have helped in improving this package.