Flutter Text Detect Area
The easy way to use this package for text recognition by selecting area over the images and live camera in Flutter. Flutter Text Detect Area's text recognition can recognize/detect text from image's particular area by dragging/moving/panning area selector. They can also be used to recognise text once and more by passing value of detect once as true/false and also can set enable/disable image interactions by passing value of enableImageInteractions.
Installing
-
Add dependency to
pubspec.yaml
Get the latest version in the 'Installing' tab on pub.dev
dependencies:
flutter_text_detect_area: <latest-version>
- Import the package
import 'package:flutter_text_detect_area/flutter_text_detect_area.dart';
Screenshot
Pick Image
You can use Image Picker
for pick image from gallery/camera to pass the image for text recognition/detection
by it's particular areas
import 'package:image_picker/image_picker.dart';
final pickedFile = await ImagePicker().pickImage(source: ImageSource.gallery);
Text Recognition/Detection Through Select area over image
After getting the picked image, we can start doing text recognition by navigate to detection screen.
Navigator.of(context).push(MaterialPageRoute(
builder: (context) => SelectImageAreaTextDetect(
detectOnce: isDetectOnce,
enableImageInteractions: enableImageInteractions,
imagePath: pickedFile?.path ?? '',
onDetectText: (v) {
setState(() {
///For single detection
if (v is String) {
detectedValue = v;
}
///For multiple area's detections
if (v is List) {
int counter = 0;
v.forEach((element) {
detectedValue += "$counter. \t\t $element \n\n";
counter++;
});
}
});
}, onDetectError: (error) {
print(error);
///This error will occurred in Android only while user will try to crop image at max zoom level then ml kit will throw max 32 height/width exception
if(error is PlatformException && (error.message?.contains("InputImage width and height should be at least 32!") ?? false)) {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(content: Text("Selected area should be able to crop image with at least 32 width and height.")));
}
},)));
Output
If you'll pass detect once as true then the result of Single Text Detection
is single dynamic value.
Screenshot
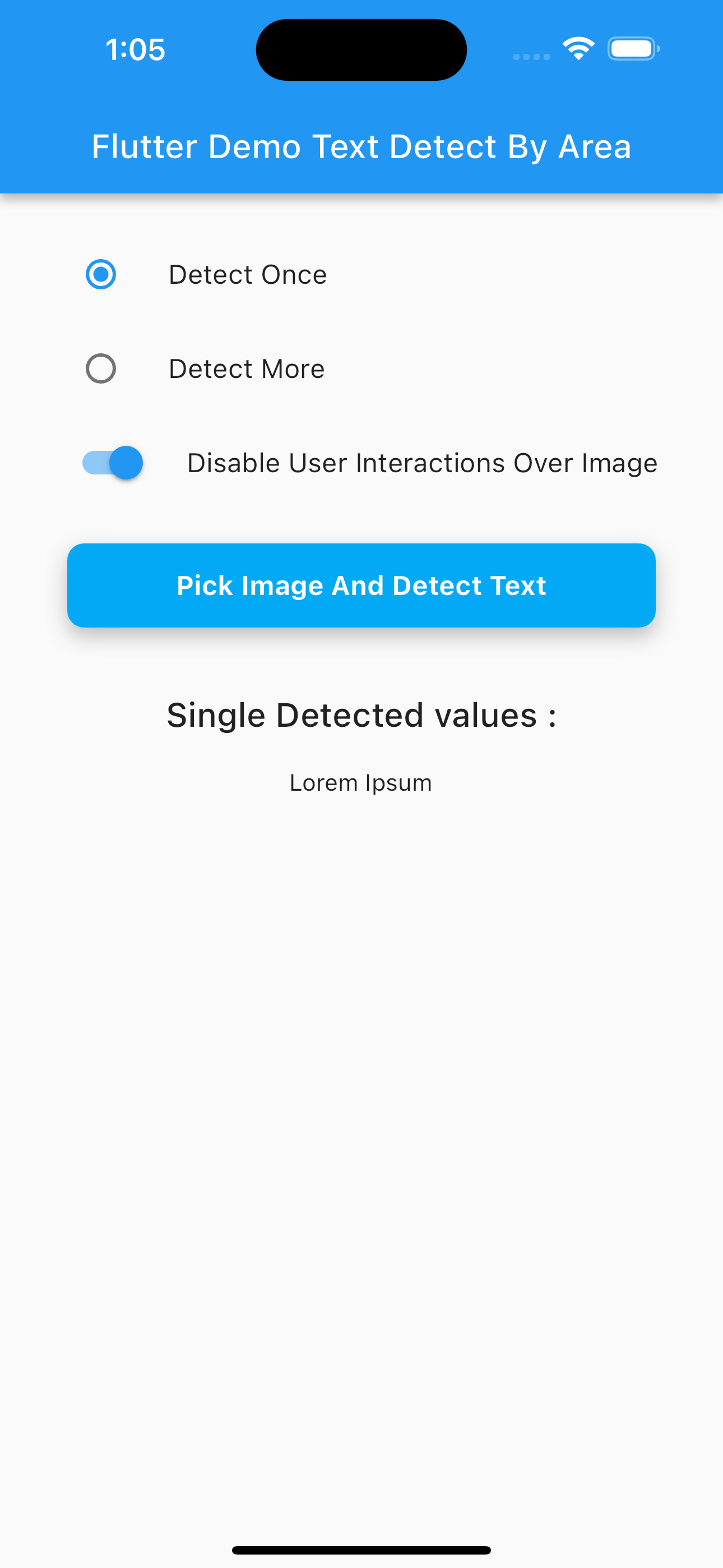
Output
If you'll pass detect once as false then the result of Multiple Text Detection Through Particular Image's Area
list of dynamic values.
Screenshot
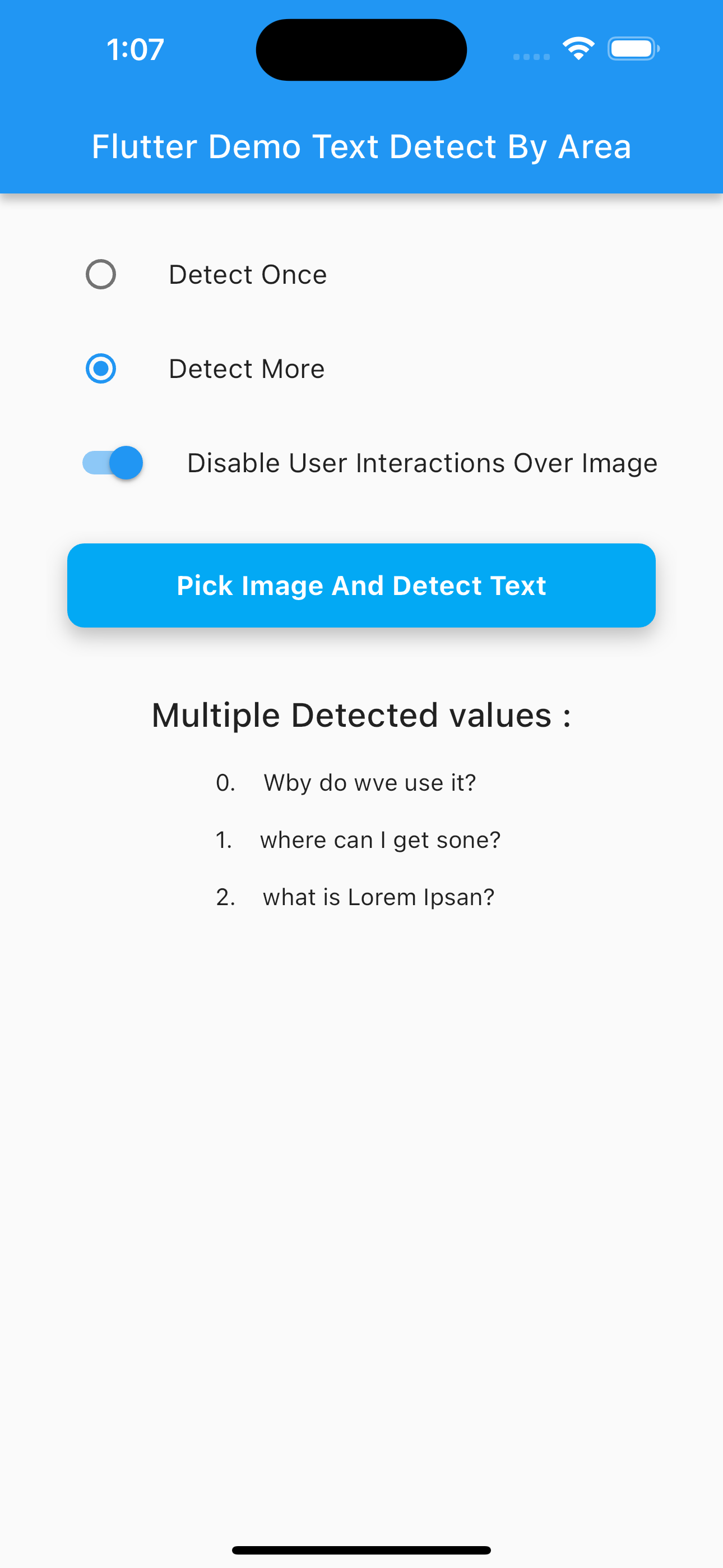
Example Project
You can learn more from example project here.
Changelog
Please see CHANGELOG for more information what has changed recently.
Main Contributors
Libraries
- flutter_text_detect_area
- FlutterTextDetectArea