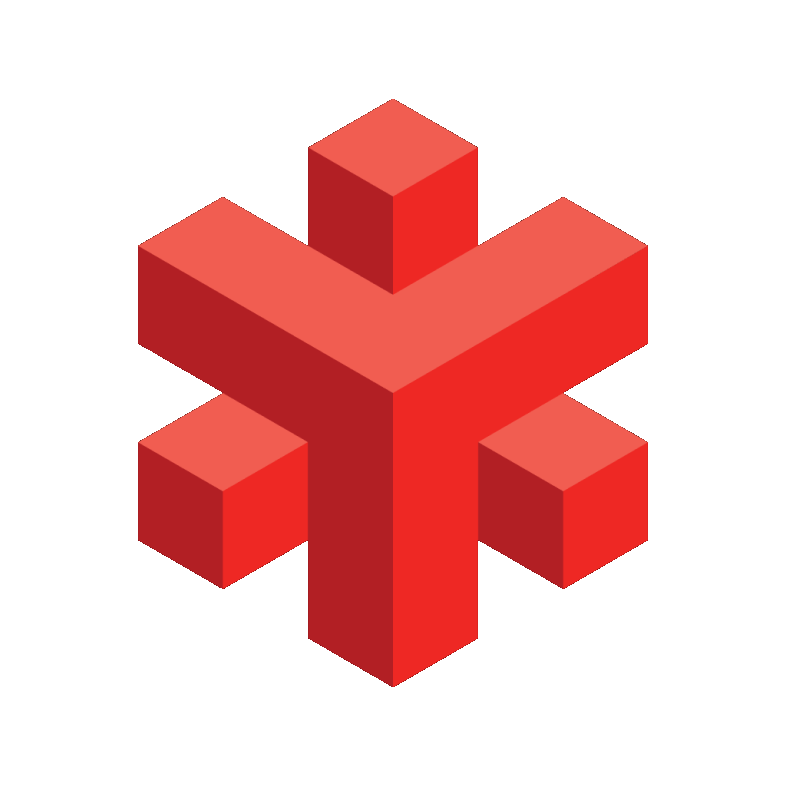
🌟 DjangoFlow Auth Flutter Package 🌟
djangoflow_auth is your ultimate Flutter authentication companion! Seamlessly integrate authentication functionalities compatible with django-df-auth. Supports multiple providers, OTP login/signup, and more, making user authentication a breeze for your Flutter app. Yes, it's ready out of the box! 🔒
NOTE: This package relies on djangoflow_openapi for models and API methods. Make sure you're using the DjangoFlow framework for development. For Django backend with DjangoFlow authentication, follow the django-df-auth README. Checkout our Example 🚀
🚀 Features 🚀
- Seamless integration with django-df-auth.
- Support for multiple authentication providers, including Google, Facebook, Apple Sign-In, Discord, and more.
- Built on top of popular and well-maintained Flutter packages like
bloc
,dio
, andhydrated_bloc
, etc. - Supports OTP, magic link, Google, Facebook, Apple, Discord for Flutter web and mobile platforms out of the box.
- Supports OAuth2 web support with
WebWindow
popup.
📑 Table of Contents 📑
📦 Installation 📦
Add the djangoflow_auth
package to your pubspec.yaml
file:
dependencies:
djangoflow_auth: <lastest_version>
dependency_overrides:
djangoflow_openapi:
path: PATH_TO_YOUR_GENERATED_OPENAPI
oauth2_client:
git:
url: https://github.com/jheld/oauth2_client.git
ref: master
Run flutter pub get
to fetch the package.
🔧 Social Login Configuration 🔧
This package has built-in support for the following social logins:- Google: djangoflow_auth_google
- Facebook: djangoflow_auth_facebook
- Apple: djangoflow_auth_apple
- Discord: djangoflow_auth_discord
- Twitter: Coming soon...
If you want to create your custom SocialLogin
, you can extend the SocialLogin
class. Here's an example from the djangoflow_auth_google
package's implementation:
class GoogleSocialLogin extends SocialLogin<GoogleSignInAccount> {
final GoogleSignIn googleSignIn;
GoogleSocialLogin({required this.googleSignIn})
: super(type: SocialLoginType.fromProvider(ProviderEnum.googleOauth2));
@override
Future<GoogleSignInAccount?> login() async {
final result = await googleSignIn.signIn();
return result;
}
@override
Future<void> logout() async {
if (await googleSignIn.isSignedIn()) {
await googleSignIn.disconnect();
}
}
}
💡 Usage 💡
Setting up AuthCubit
- Import the necessary packages, for example using BlocProvider:
```dart
import 'package:flutter_bloc/flutter_bloc.dart';
import 'package:djangoflow_auth/djangoflow_auth.dart';
import 'package:djangoflow_auth_google/djangoflow_auth.dart_google';
import 'package:google_sign_in/google_sign_in.dart';
```
- Initialize `AuthCubit` and provide social login providers via `BlocProvider`
```dart
void main() {
runApp(
BlocProvider<AuthCubit>(
create: (context) => AuthCubit.instance
..authApi = {Your AuthApi, comes from djangoflow_openapi}
..socialLogins = [
GoogleSocialLogin(
type: SocialLoginType.fromProvider(
ProviderEnum.googleOauth2,
),
googleSignIn: GoogleSignIn(
scopes: [
'email',
],
),
),
// Add other social login providers
],
child: MyApp(),
),
);
}
```
Performing OTP Login
Inside your app, use the AuthCubit
to perform OTP login:
final authCubit = context.read<AuthCubit>();
try {
// Step 1: Request OTP for the user's email
await authCubit.requestOTP(email: userEmail);
// Step 2: Present the OTP input UI to the user and retrieve the OTP value
// Step 3: Initiate OTP login
await authCubit.loginWithEmailOTP(email: userEmail, otp: enteredOTP);
// Step 4: Handle successful login and proceed with app navigation
} catch (error) {
// Handle errors, e.g., display an error message to the user
}
Performing magic link login
Inside your app, use the AuthCubit to perform login with a Magic Link:
final authCubit = context.read<AuthCubit>();
try {
// Step 1: Retrieve the magic link from your user's source (e.g., email + otp) in base64
final magicLink = "your_encoded_magic_link_here"; // Replace with the actual magic link
// Step 2: Initiate login with the user's email and OTP
await authCubit.loginWithMagicLink(magicLink: magicLink);
// Step 3: Handle successful login and proceed with app navigation
} catch (error) {
// Handle errors, e.g., display an error message to the user
}
Performing Social Authentications
Let's say we are going to login via google, inside your app, initiate social login using AuthCubit
methods
-
Add the
djangoflow_auth_google
package to yourpubspec.yaml
file:dependencies: djangoflow_auth_google: <latest_version> google_sign_in: <latest_version>
and run
flutter pub get
-
Then when you need to authenticate with Google sign in,
final authCubit = context.read<AuthCubit>(); final socialLogin = authCubit.socialLogins.getSocialLoginByProvider( ProviderEnum.googleOauth2, ); // Authenticate with Google final result = await authCubit.requestTokenFromSocialProvider< GoogleSignInAccount>( socialLogin.type, );
Handling Social Login Results
Based on the login result, retrieve the access token and use it to log in with the social provider:
if (result == null) {
throw Exception('Google Sign In failed');
} else {
final googleSignInAuthentication =
await result.authentication;
final accessToken =
googleSignInAuthentication.accessToken;
if (accessToken == null) {
throw Exception(
'Google Sign In failed - no token');
}
await authCubit.loginWithSocialProvider(
socialTokenObtainRequest:
SocialTokenObtainRequest(
provider: socialLogin.type.provider,
accessToken: accessToken,
),
);
}
🌟 Example 🌟
👏 Contributions and Issues 👏
Contributions, bug reports, and feature requests are welcome! Feel free to submit a pull request or open an issue on the GitHub repository.
📄 License 📄
This package is distributed under the MIT License.