Add to Gallery
Adds images and videos to the Android Gallery and iOS Photos
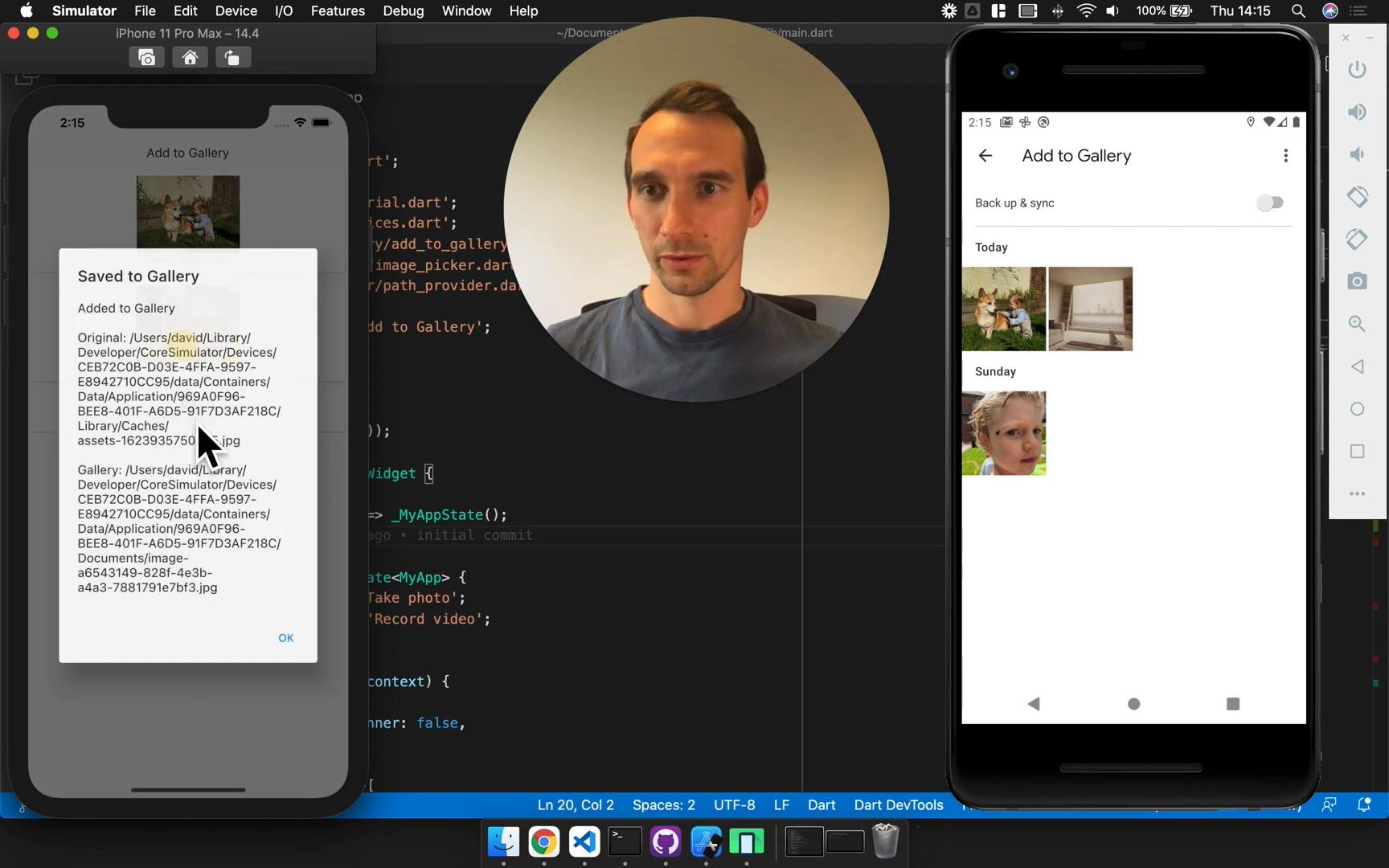
View example app
Installation
Add add_to_gallery
as a dependency in your pubspec.yaml file.
Permissions
You need the following permissions in your app:
- iOS
NSPhotoLibraryUsageDescription
- This allows the plugin to use the PHPhotoLibrary APIs to add assets to the user's photo library.
- Android
READ_EXTERNAL_STORAGE
WRITE_EXTERNAL_STORAGE
- If you target Android 10, you will also need the requestLegacyExternalStorage="true" in your AndroidManifest.
This plugin does not manage permissions for you. By excluding permissions from our plugin we have created a simple, reliable plugin.
We recommend using permission_handler to handle permissions.
Usage
There's only one method. It copies the source file to the gallery and returns the new file.
File file = await AddToGallery.addToGallery(
originalFile: File('/Some/Media/Path.jpg'),
albumName: 'My Awesome App',
deleteOriginalFile: false,
);
print("Savd to gallery with Path: ${file.path}");
Using permission_handler, this may look like:
try {
// iOS
if (!await Permission.photos.request().isGranted) {
throw ('Permission Required');
}
// Android (10 and below)
if (!await Permission.storage.request().isGranted) {
throw ('Permission Required');
}
// Add to the gallery
File file = await AddToGallery.addToGallery(
originalFile: File('/Some/Media/Path.jpg'),
albumName: 'My Awesome App',
deleteOriginalFile: true,
);
print("Savd to gallery with Path: ${file.path}");
} catch(e) {
print("Error: $e");
}
Example app
The example app shows a few more edge-cases in action.
- Uses permission_handler to request permissions
- Uses image_picker to take photos with the camera
- Copies assets to the gallery
- Uses shared_preferences to save and read the file path locally
- This shows that the assets are still accessible between reboots
Credits & Comparison
Add to Gallery is based on gallery_saver with some notable differences. Enough to warrant a new package rather than a pull-request. Generally speaking, I've simplified the API and unified the behaviour on iOS and Android. It also supports scoped storage on Android (which will be enforced with Android 11)
Big thanks to the Tecocraft LTD team for Android functionality on Android 10 and 11.
Feature |
gallery_saver
original package |
add_to_gallery
this package |
---|---|---|
General Behaviour |
Android
|
Android and iOS
|
Return Value | Returns bool for the success of the operation
|
Returns a File pointing to the new file in the gallery |
Remote Files | Automatically downloads files that start with http | Does not download files that start with http |
Album Name | Optional with default values | Is required |
Image Manipulation |
Android
|
Does not manipulate images |
Permissions | Handled within the plugin. The first-call fails silently due to permissions race-condition | Not handled within the plugin |
An Important Note about Google photos
Google Photos has a built-in feature to remove exact duplicates. It can be confusing to see your media disappearing like this. I considered addressing this behaviour in the plugin, but decided against it. I expect plugin users to be creating unique images with the camera or other methods.