This Flutter package provides functionalities to retrieve and manage YouTube video information, including details about the video and the uploader, as well as various thumbnail resolutions.
Configuration
Before fetching video details, you need to configure the package with the video ID:
await Youtube.config(videoId: 'your_video_id');
Accessing Video Information
You can access the video and channel details using the provided getters:
var channelDetails = Youtube.channelDetails;
var videoDetails = Youtube.videoDetails;
var thumbnails = Youtube.thumbnails;
ChannelDetails
Holds details about the YouTube channel.
-
Properties
- String
name
: The name of the uploader. - String
id
: The ID of the channel. - String
username
: The username of the uploader.
- String
-
Example
var channelDetails = Youtube.channelDetails;
print('Channel Name: ${channelDetails.name}');
print('Channel ID: ${channelDetails.id}');
print('Channel Username: ${channelDetails.username}');
VideoDetails
Holds details about the YouTube video.
-
Properties
- String
title
: The title of the video. - int
duration
: The duration of the video in seconds. - int
viewCount
: The view count of the video. - String
defaultThumbnail
: The URL of the default thumbnail.
- String
-
Example
var videoDetails = Youtube.videoDetails;
print('Video Title: ${videoDetails.title}');
print('Video Duration: ${videoDetails.duration} seconds');
print('Video View Count: ${videoDetails.viewCount}');
print('Default Thumbnail: ${videoDetails.defaultThumbnail}');
Thumbnails
Holds URLs for different resolutions of the video's thumbnails.
-
Properties
- String
fullhd
: URL for the Full HD thumbnail. - String
hd
: URL for the HD thumbnail. - String
sd
: URL for the SD thumbnail. - String
hq
: URL for the HQ thumbnail. - String
lq
: URL for the LQ thumbnail.
- String
-
Example
var thumbnails = Youtube.thumbnails;
print('Full HD Thumbnail: ${thumbnails.fullhd}');
print('HD Thumbnail: ${thumbnails.hd}');
print('SD Thumbnail: ${thumbnails.sd}');
print('HQ Thumbnail: ${thumbnails.hq}');
print('LQ Thumbnail: ${thumbnails.lq}');
Video Infortimation 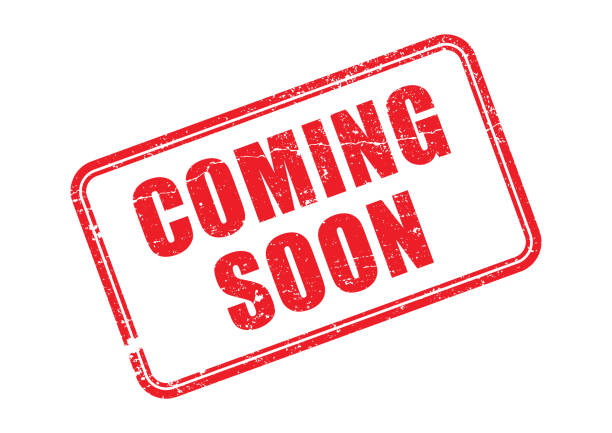
- String
url
- URL of the video for allquality with direct streaming and downloading - int
fps
- Frames per second - String
resolution
- Resolution of the video - String
audio
- Audio of the video - int
filesize
- Filesize of the video - String
quality
- Quality of the video - String
videoExtension
- Video extension - int
height
- Height of the video - int
width
- Width of the video
Example
👉 For a complete example, refer to the Youtube package documentation.
Report bugs or issues
You are welcome to open a ticket on github if any 🐞 problems arise. New ideas are always welcome.
Note: This package uses third-party api to fetch data.