Sn Progress Dialog
Progress dialog package for flutter
Getting Started
You must add the library as a dependency to your project.
dependencies:
sn_progress_dialog: ^1.1.4
You should then run flutter packages get
Now in your Dart code, you can use:
import 'package:sn_progress_dialog/sn_progress_dialog.dart';
Normal Progress | Valuable Progress |
---|---|
![]() |
![]() |
Preparing Progress | Custom Progress |
---|---|
![]() |
![]() |
Usage Example
Create Progress Dialog
ProgressDialog pd = ProgressDialog(context: context);
Set options
- Both parameters are not mandatory with version 1.1.3 (max, msg)
- Default values can be changed according to the intended use.
pd.show(max: 100, msg: 'File Downloading...');
You don't need to update state, just pass the value.
- If you're not interested in showing value, you don't need to update progressValue.
pd.update(value: ProgressValue, msg: "Updated message");
Completed Type
- Use this to update the dialog when the process is finished. If it is Empty, progress automatically closes.
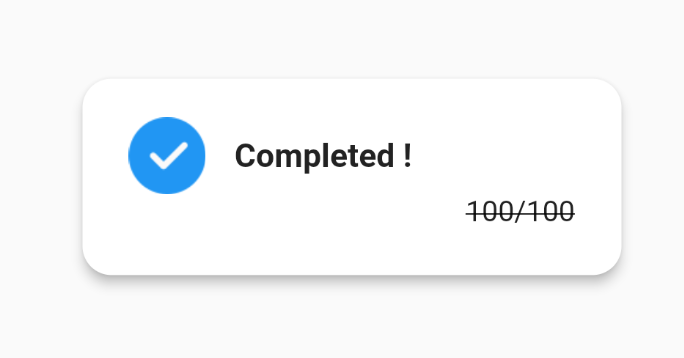
completed: Completed() // To use with default values
completed: Completed(completedMsg: "Downloading Done !", completedImage: AssetImage("image path"), completionDelay: 2500)
Cancel Option
- Use the "cancel" class to create a cancel button
cancel: Cancel() // To use with default values
cancel: Cancel(cancelImageSize: 20.0, cancelImageColor: Colors.blue, cancelImage: AssetImage("image path"), cancelClicked: () {})
Dialog Status
- Returns the current state of the dialog.
onStatusChanged: (status) {
if (status == DialogStatus.opened)
print("opened");
else if (status == DialogStatus.closed)
print("closed");
else if (status == DialogStatus.completed)
print("completed");
}
Other Properties
Dialog closes automatically when its progress status equals the max value. Use this method if you want to turn it close manually.
pd.close();
Returns whether the dialog box is open.
pd.isOpen();
Example Use With Dio
var dio = new Dio();
ProgressDialog pd = ProgressDialog(context: context);
pd.show(max: 100, msg: 'File Downloading...');
await dio.download(
'your download_url',
'your path',
onReceiveProgress: (rec, total) {
int progress = (((rec / total) * 100).toInt());
pd.update(value: progress);
},
);