π Language: English | δΈζ
Show Touches
Show finger touch effects for Flutter!
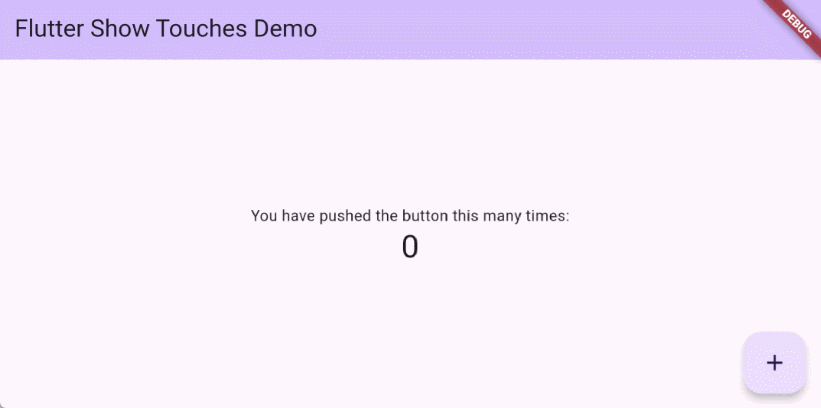
Table of contents πͺ
Features β¨
- π Multiple fingers (pointers)
- π§ Controller control
- βοΈ Customizable pointer widgets and animations
Install π―
Versions compatibility π¦
Flutter | 3.3.0+ |
---|---|
show_touches 0.0.1+ | β |
Platforms compatibility π±
Android | iOS | Web | macOS | Windows | Linux |
---|---|---|---|---|---|
β | β | β | β | β | β |
Add package π¦
Run this command with Flutter,
$ flutter pub add show_touches
or add show_touches
to pubspec.yaml
dependencies manually.
dependencies:
show_touches: ^latest_version
Simple usage π
Example: show_touches/example
ShowTouches π¦
ShowTouches widget will have default gesture logic and pointer widgets.
/// Import show_touches
import 'package:show_touches/show_touches.dart';
MaterialApp(
builder: ShowTouches.init(),
home: XxxPage(),
);
/// or
ShowTouches(child: XxxPage()),
Usage π
ShowTouches
widget parameters π€
Parameter | Type | Default | Description |
---|---|---|---|
child required |
Widget |
- | - |
enable | bool |
true |
true (enable) false (disable) |
controller | ShowTouchesController? | null |
ShowTouchesController to control the pointer. |
pointerBuilder | PointerBuilder? | null |
Custom pointer widget, but it will cause the defaultPointerStyle to be invalid. |
defaultPointerStyle | DefaultPointerStyle |
DefaultPointerStyle() |
Default style for the pointer widget when pointerBuilder is not used. |
showDuration | Duration |
Duration(milliseconds: 50) |
Show animation duration (pointer). |
removeDuration | Duration |
Duration(milliseconds: 200) |
Remove animation duration (pointer). |
PointerBuilder π
Example:
ShowTouches(
pointerBuilder: (
BuildContext context,
int pointerId,
Offset position,
Animation<double> animation,
) {
final Animation<double> scaleAnimation = Tween<double>(
begin: 2.0,
end: 1.0,
).animate(animation);
return Positioned(
left: position.dx - 30.0,
top: position.dy - 30.0,
child: IgnorePointer(
ignoring: true,
child: ScaleTransition(
scale: scaleAnimation,
child: FadeTransition(
opacity: animation,
child: Container(
width: 60,
height: 60,
color: Colors.black,
),
),
),
),
);
},
child: XxxPage(),
),
Parameter | Type | Description |
---|---|---|
context | BuildContext |
- |
pointerId | int |
Pointer (touch) ID. |
position | Offset |
Current touch position. |
animation | Animation<double> |
Animation controller. |
ShowTouchesController π
You can use the controller to control the pointers in the ShowTouches
widget,
or you can implement your own gesture logic to control the pointers.
Example:
final ShowTouchesController controller = ShowTouchesController();
......
@override
void dispose() {
controller.dispose();
super.dispose();
}
......
Listener(
onPointerDown: (event) => controller.addPointer(...),
onPointerMove: (event) => controller.updatePointer(...),
onPointerUp: (event) => controller.removePointer(...),
onPointerCancel: (event) => controller.removePointer(...),
behavior: HitTestBehavior.translucent,
child: child,
);
Get all pointer data
controller.data
to get all the pointer data (Map<int, PointerData>
).
Map<int, PointerData> ->
key
: pointerId,value
: PointerData
PointerData
Parameter | Type | Description |
---|---|---|
pointerId | int |
Pointer (touch) ID. |
positionState | ValueNotifier<Offset> |
Current touch position. |
animationController | AnimationController |
Animation controller. |
pointerOverlayEntry | OverlayEntry? |
Pointer OverlayEntry . |
addPointer()
Parameter | Type | Default | Description |
---|---|---|---|
context required |
BuildContext |
- | - |
pointerId required |
int |
- | Pointer (touch) ID. |
position required |
Offset |
- | Current touch position. |
animationController required |
AnimationController |
- | Animation controller. |
pointerBuilder | PointerBuilder? |
null |
Custom pointer widget, but it will cause the defaultPointerStyle to be invalid. |
defaultPointerStyle | DefaultPointerStyle |
DefaultPointerStyle() |
Default style for the pointer widget when pointerBuilder is not used. |
updatePointer()
Parameter | Type | Default | Description |
---|---|---|---|
pointerId required |
int |
- | Pointer (touch) ID. |
position required |
Offset |
- | Current touch position. |
removePointer()
Parameter | Type | Default | Description |
---|---|---|---|
pointerId required |
int |
- | Pointer (touch) ID. |
disposePointer()
Parameter | Type | Default | Description |
---|---|---|---|
pointerId required |
int |
- | Pointer (touch) ID. |
Contributors β¨
AmosHuKe |
License π
Open sourced under the MIT license.
Β© AmosHuKe
Libraries
- show_touches
- Show finger touch effects for Flutter, with support for multiple fingers, controllers, custom widgets, and animations.