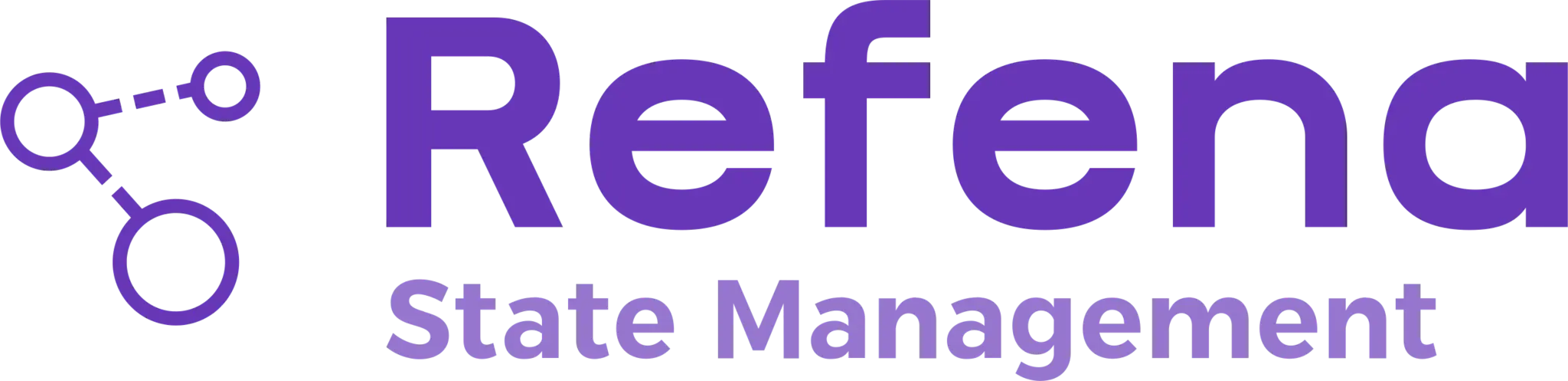
Provides extension getters to use Riverpod and Refena at the same time.
Checkout Refena for Riverpod developers for more information.
// Riverpod -> Refena
ref.refena.read(myRefenaProvider);
// Refena -> Riverpod
ref.riverpod.read(myRiverpodProvider);
Usage
➤ Setup
Add the following dependencies to your pubspec.yaml
:
# pubspec.yaml
dependencies:
flutter_riverpod: <version>
refena_flutter: <version>
refena_riverpod_extension: <version>
Wrap your app with RefenaRiverpodExtension
(below RefenaScope
and ProviderScope
):
void main() {
runApp(
ProviderScope(
child: RefenaScope(
child: RefenaRiverpodExtension(
child: MyApp(),
),
),
),
);
}
➤ Access Riverpod from Refena
Let's say you have a StateProvider
written in Riverpod:
final riverpodCounterProvider = StateProvider((ref) => 0);
Then you can access it from Refena by using the Ref.riverpod
getter:
final refenaProvider = ViewProvider((ref) {
// This is reactive!
// The refenaProvider will be rebuilt when the riverpodCounterProvider changes.
final counter = ref.riverpod.watch(riverpodCounterProvider);
return counter.state;
});
➤ Access Refena from Riverpod
Let's say you have a ReduxProvider
written in Refena:
final counterProvider = ReduxProvider<Counter, int>((ref) => Counter());
class Counter extends ReduxNotifier<int> {
@override
int init() => 10;
}
class AddAction extends ReduxAction<Counter, int> {
final int amount;
AddAction(this.amount);
@override
int reduce() => state + amount;
}
Then you can access it by using the Ref.refena
getter:
final riverpodProvider = Provider((ref) {
// There is no reactive way to access Refena from Riverpod.
return ref.refena.read(counterProvider);
});
final riverpodProvider2 = NotifierProvider<RiverpodCounter, int>(() {
return RiverpodCounter();
});
class RiverpodCounter extends Notifier<int> {
@override
int build() => 0;
void dispatch(int amount) {
// We can dispatch actions to Refena from Riverpod.
ref.refena.redux(counterProvider).dispatch(AddAction(amount));
}
}