Welcome to prep
Inspired by the C preprocessor, this package helps you manage your project with unique source code expressions. It also comes with the PrepLog class that's an ideal tool for logging and debugging.
This package includes a compilable CLI application that can be used to prep source files of any programming language. See source code on GitHub: https://github.com/robmllze/prep.
The CLI works well with any Dart project including Flutter web projects.
Example
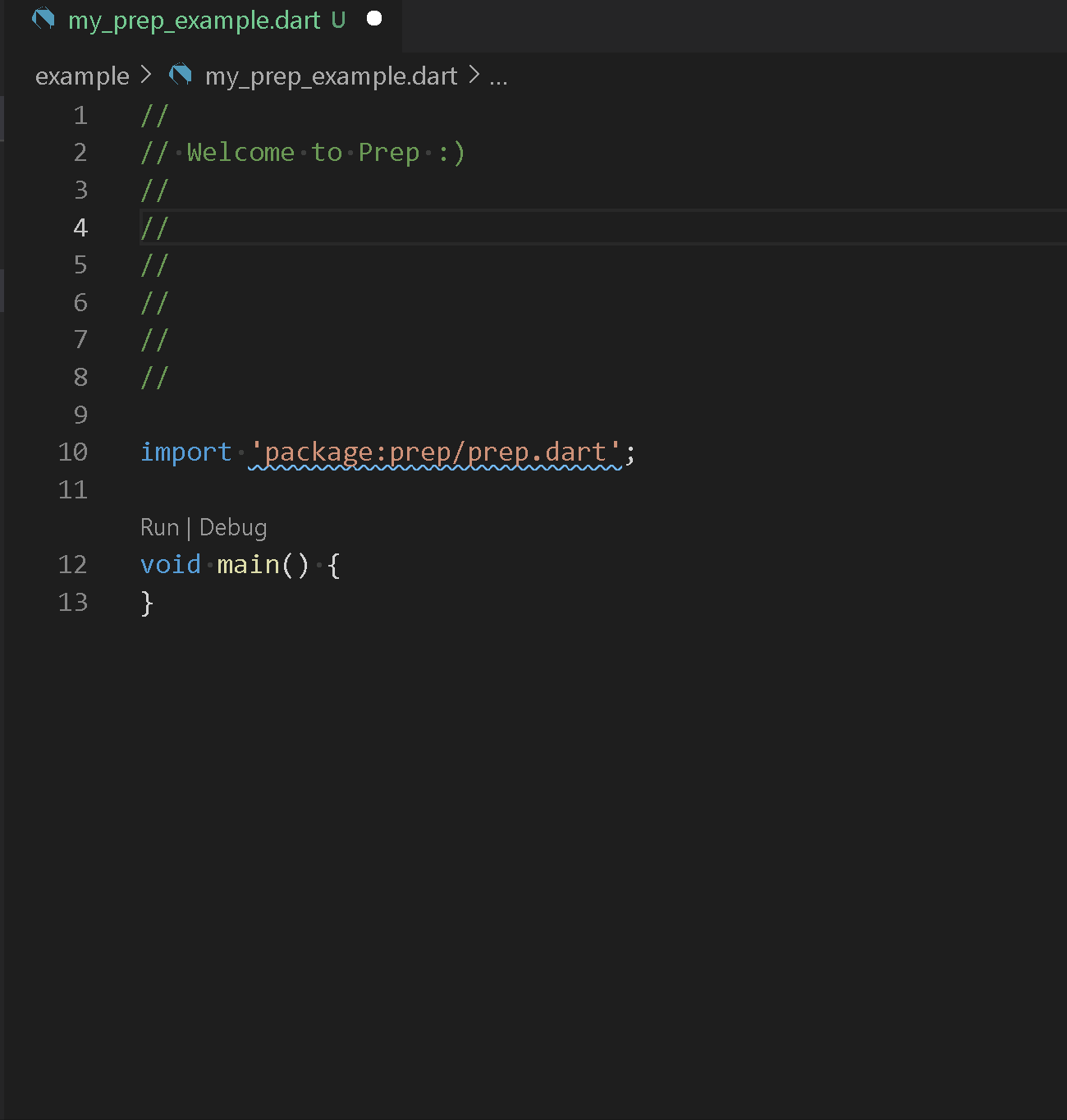
Basics
1/3 - Examine the following code (prep_example.dart):
// <##Title>
// <#Author=>
// <#Date=>
// <#Time=>
import 'package:prep/prep.dart';
const _LOG = PrepLog.file("<#f=>");
void main() {
// Process source files as per prep.yaml.
prep();
print("Follow me on Instagram " + "<#Instagram=>".prepValue);
print("This file is " + "<#f=>".prepValue);
print("This line is number " + "<#l=>".prepValue);
// Use double ## to completely replace.
print("And this line is number " + "<##l>");
print("The time now is " + "<#t>".prepValue);
// Package as per pubspec.yaml.
print("This package is " + "<#Package>".prepValue);
// Version as per pubspec.yaml.
print("The package version is " + "<#Version>".prepValue);
print("Let's print the USERNAME environment variable: " +
"<#ENV USERNAME=>".prepValue);
if (int.tryParse("42") == 42) {
// PrepLog is a great tool for debugging!
_LOG.note(
"The answer to life, the Universe and everything is...42!",
"<#l=>",
);
}
}
2/3 - After running the code above, it will be modified to this:
// PREP EXAMPLE
// <#Author = Robert Mollentze>
// <#Date = 8/26/2021>
// <#Time = 23:15>
import 'package:prep/prep.dart';
const _LOG = PrepLog.file("<#f=prep_example.dart>");
void main() {
// Process source files as per prep.yaml.
prep();
print("Follow me on Instagram " + "<#Instagram = @robmllze>".prepValue);
print("This file is " + "<#f=prep_example.dart>".prepValue);
print("This line is number " + "<#l=15>".prepValue);
// Use double ## to completely replace.
print("And this line is number " + "17");
print("The time now is " + "<#t=23:15>".prepValue);
// Package as per pubspec.yaml.
print("This package is " + "<#Package = prep>".prepValue);
// Version as per pubspec.yaml.
print("The package version is " + "<#Version = 0.3.5>".prepValue);
print("Let's print the USERNAME environment variable: " +
"<#ENV USERNAME = guest>".prepValue);
if (int.tryParse("42") == 42) {
// PrepLog is a great tool for debugging!
_LOG.note(
"The answer to life, the Universe and everything is...42!",
"<#l=29>",
);
}
}
3/3 - Finally, this will be the output:
Follow me on Instagram @robmllze
This file is prep_example.dart
This line is number 13
And this line is number 15
The time now is 23:15
This package is prep
The package version is 0.3.5
Let's print the USERNAME environment variable: guest
[0] 🟢 In FILE prep_example.dart and LINE 27 ⏳ 0.003s
"The answer to life, the Universe and everything is...42!"
Expressions
Current Time:
Update: <#Time= >
or <#t= >
Replace: <##Time>
or <##t>
Relative Path of Current File:
Update: <#Path= >
or <#p= >
Replace: <##Path>
or <##p>
Short File Name of Current File:
Update: <#File= >
or <#f= >
Replace: <##File>
or <##f>
Current Line:
Update: <#Line= >
or <#l= >
Replace: <##Line>
or <##l>
Operating System:
Update: <#OS= >
Replace: <##OS>
File Title:
Update: <#Title= >
Replace: <##Title>
Environment Variables:
Update: <#ENV PATH= >
or <#ENV COMPUTERNAME= >
or <#ENV USERNAME= >
etc.
Replace: <##ENV PATH>
or <##ENV COMPUTERNAME>
or <#ENV USERNAME>
etc.
Comment Breaks:
// <##br-heavy>
// <##br-medium>
// <##br-light>
// <##br-dash>
// <##br-line>
Intro:
// <##intro>
Installing
dev_dependencies:
prep: ^0.3.5 # https://pub.dev/packages/prep
Configuring
Add the following to prep.yaml in your project's root directory:
# This file was auto-generated by prep().
# Rename it to "prep.yaml" to use it.
# If you like this package, please follow me on Instagram @robmllze.
# Feel free to DM me about bugs or questions.
# Files in this directory and all its subdirectories will be parsable.
path: "."
# If you need to tweak the syntax, do it here.
# Note: This is experimental!
syntax_beg: "<" # This also works well: "`"
syntax_end: ">" # This also works well: "`"
syntax_sep: "=" # This also works well: ":"
# Set to true to include your environment variables.
# Note: This significantly slows down the prep function but it does
# not matter if you use it asynchronously.
include_env: true
# Specify which custom fields to update.
update_these_fields: {
"Author": "Robert Mollentze",
"GitHub": "@robmllze",
"Instagram": "@robmllze",
"Email": "robmllze@gmail.com",
}
# Specify which file types to parse.
parse_these_file_types: [
"dart",
"md",
"yaml",
]
# Specify which files not to parse.
dont_parse_these_files: [
"prep.yaml",
"README.md",
]
Alternatively, you can specify configurations in the prep function. This will override whatever's in prep.yaml.
void main() {
// ...
prep(
path: ".",
parseTheseFileTypes: [
"dart",
"yaml",
],
dontParseTheseFiles: [
"prep.yaml",
],
updateTheseFields: {
"Author": "Robert Mollentze",
"GitHub": "@robmllze",
"Instagram": "@robmllze",
"Email": "robmllze@gmail.com",
},
includeEnv: true,
);
// ...
}
Finally
Have a peak at my other package, elliptic_text, available at https://pub.dev/packages/elliptic_text/.
Please let me know if you find any bugs or if you have suggestions.
Thanks,
Robert Mollentze
GitHub: https://github.com/robmllze/
Medium: https://robmllze.medium.com/
Email: robmllze@gmail.com
Libraries
- prep
- Inspired by the C preprocessor, this package helps you manage your project with unique source code expressions.