Phone Verification
This package helps the flutter developer to send OTP to the user and verify user's Phone Number.
βIt is recommended to use this package with real device and not emulator
β Currently it only works on Android.
Features
π Free OTP Verification System.
π Reliable
π 4 digit OTP
Demo
π I am hide my number due to privacy issue
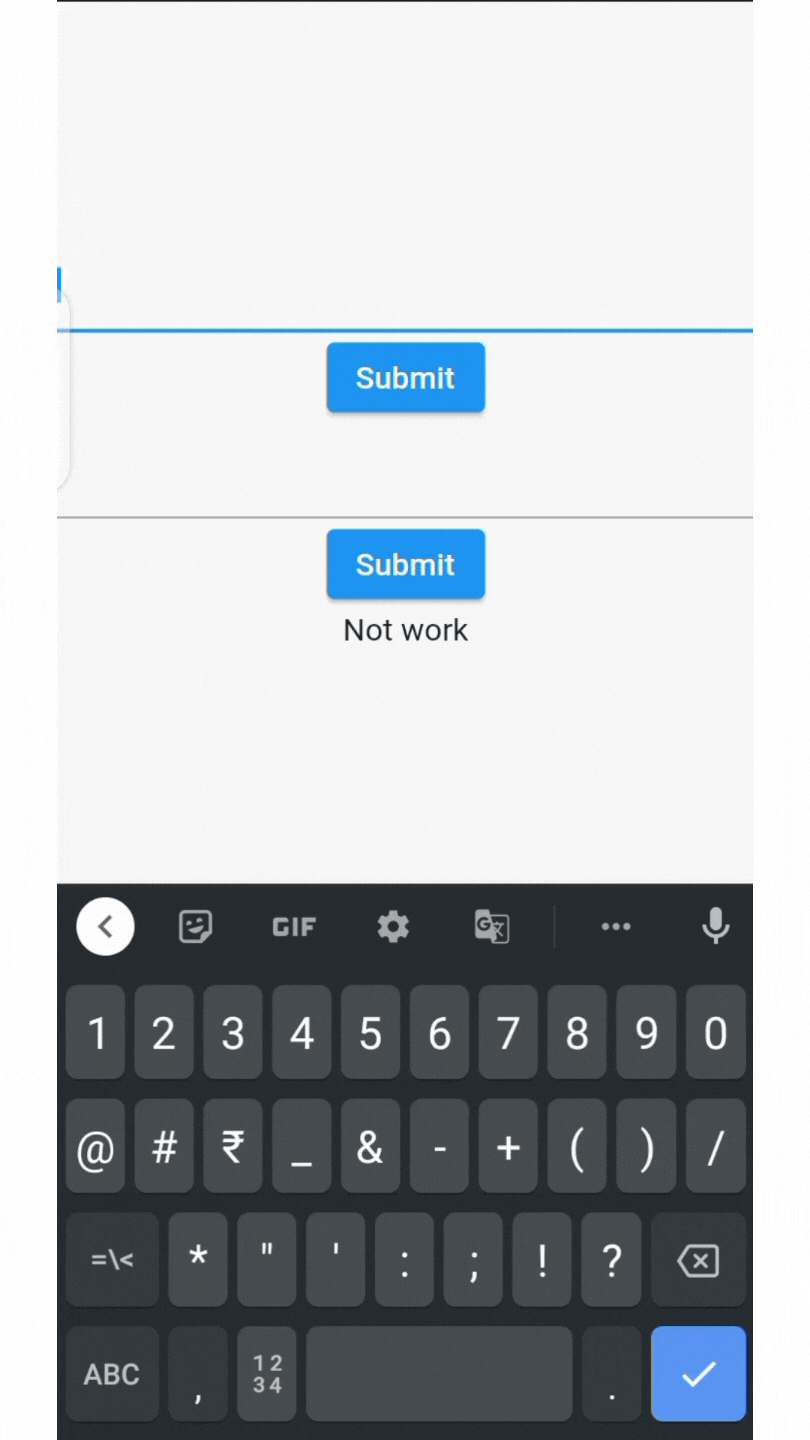
Android
- Inside
android/app/build.gradle
file change following sdks
compileSdkVersion 31 //change to 31
defaultConfig {
--------------------------------------
--------------------------------------
minSdkVersion 21 //change to 21
targetSdkVersion 30 //change to 30
--------------------------------------
--------------------------------------
}
- Inside
android/app/src/main/AndroidManifest.xml
add the following two lines
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.mysql_register">
<!-- add these lines π -->
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.SEND_SMS" />
<!-- add these lines π -->
<application
Installation
- First, add the latest
phone_verification
package to your pubspec dependencies.
dependencies:
phone_verification: ^0.0.1
- Second import package to your file
import 'package:phone_verification/phone_verification.dart'
- Call the Instance of PhoneVerification class
PhoneVerification phoneverification = PhoneVerification(number: usernumber);
//here usernumber is the Phone number which is to be verified
Send OTP
π In the following codes usernumber should be use without country code and Your Otp is the message I want to send with the OTP, you can change that according to your choice.
PhoneVerification phoneverification = PhoneVerification(number:'93514xxxxx' );
phoneverification.sendotp('Your Otp');
or
PhoneVerification(number: '93514xxxxx').sendotp('Your Otp');
Verify OTP
π Pass the OTP entered by the user to verifyotp() method here enteredotp
is the user typed OTP.
String check= 'No acion';
ElevatedButton(
onPressed: () async {
//write the below line to verify
bool verify = await PhoneVerification(number: '93514xxxxx').verifyotp(enteredotp.toString());
//if verified successfully it will return true else return false
if (verify == true) {
check = 'Verify';
}
else {
check = 'error';
}
},
child: Text("Tap To Verify")),
or
String check= 'No acion';
ElevatedButton(
onPressed: () async {
//write the below line to verify
PhoneVerification phoneverification = PhoneVerification(number: '93514xxxxx');
bool verify = await phoneverification.verifyotp(enteredotp.toString());
//if verified successfully it will return true else return false
check = verify == true ? 'verify' : 'error';
}
child: Text("Tap To Verify")),