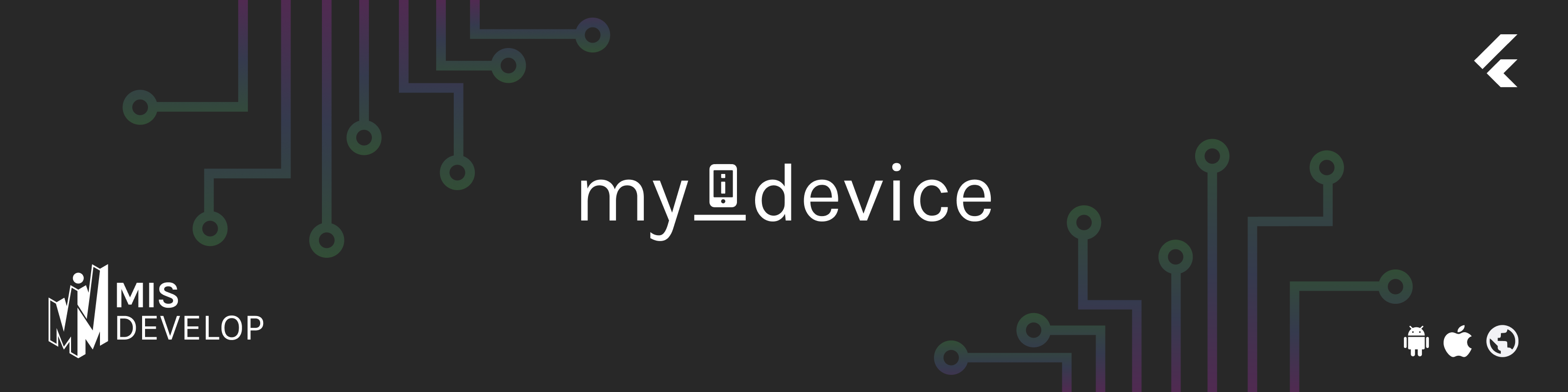
Features
Agile & easy handler for device information đą
Includes Device Info Plus âšī¸ and Connectivity Plus đ implementations
Getting started
In the pubspec.yaml
of your flutter project, add the following dependency:
dependencies:
...
my_device: ^0.2.0
In your library add the following import:
import 'package:my_device/my_device.dart';
Once context is initialized, do the following to initialize the DeviceManager instance:
DeviceManager(context);
You can also use the configure method to initialize the DeviceManager instance, responsive breakpoints and Connectivity configuration:
DeviceManager.configure(
context: context,
responsiveBreakpoints: const ResponsiveBreakpoints(
mobile: 400, //Default is 500
desktop: 1000, //Default is 900
),
connectivityConfigure: ConnectivityConfigure(
onConnectivityChanged: (connectivity) {
print('Connectivity changed: $connectivity');
},
onConnectionLost: () {
print('Connection lost');
},
onConnectionReestablished: () {
print('Connection reestablished');
},
useDefaultBehaviour: true // Shows a default toast when set to true
),
);
// Mobile view < mobile
// Tablet view > mobile && < desktop
// Desktop view > desktop
Usage
Any method can be accessed through the DeviceManager instance or through the context
extension
Getters for screen height and width
final screenHeight = DeviceManager.screenHeight;
final screenWidth = DeviceManager.screenWidth;
final height = DeviceManager.height;
final width = DeviceManager.width;
final heightFromContext = context.height;
final widthFromContext = context.width;
Getters for responsive sizes (dependent of the configured responsive breakpoints)
final isPhoneView = DeviceManager.isPhoneView; // width < mobile
final isTabletView = DeviceManager.isTabletView; // mobile <= width < desktop
final isDesktopView = DeviceManager.isDesktopView; // width >= desktop
Simple methods for platform information
final isWeb = DeviceManager.isWeb;
final isAndroid = DeviceManager.isAndroid;
final isIOS = DeviceManager.isIOS;
final isMacOS = DeviceManager.isMacOS;
final isLinux = DeviceManager.isLinux;
final isWindows = DeviceManager.isWindows;
final platformName = DeviceManager.platformName;
final platformIcon = DeviceManager.platformIcon;
Direct method for device default padding
final padding = DeviceManager.viewPadding;
final paddingFromContext = context.viewPadding;
Connectivity Plus methods
Get the current status
final connectivity = DeviceManager.connectivity;
final connectivityFromContext = context.connectivity;
Listen on connectivity changes
DeviceManager.listenConnectivityChanges();
Set any custom connectivity method after configure callback
DeviceManager.onConnectionLost = () {
print('Connection lost');
};
DeviceManager.onConnectionReestablished = () {
print('Connection reestablished');
};
DeviceManager.onConnectivityChanged = (connectivity) {
print('Connectivity changed: $connectivity');
};
Device Info Plus shortened method
final deviceInfo = DeviceManager.deviceInfo;
Additional information
This package assumes corresponding permissions depending on platform. Also take a look at the example app for further information.