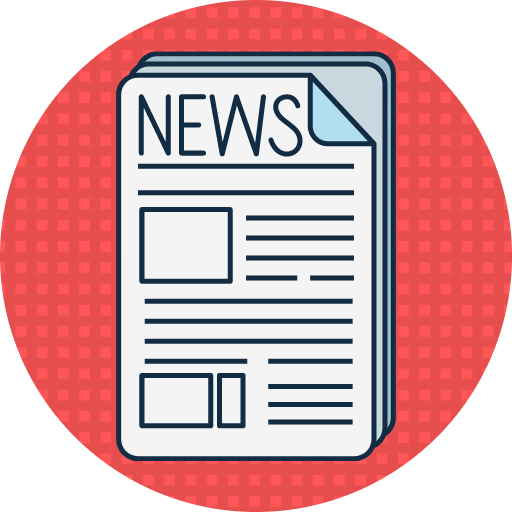
InShorts Flutter API
Get access to inshorts app's private API in flutter
🌈Categories
- All News
- Trending
- Top Stories
- National
- Business
- Politics
- Sports
- Technology
- Startups
- Entertainment
- Hatke
- Education
- World
- Automobile
- Science
- Travel
- Miscellaneous
- Fashion
🔥 Usage
Add the dependency in pubspec.yaml
:
dependencies:
...
inshorts_flutter: latest_version
Get news
import 'package:flutter/material.dart';
import 'package:inshorts_flutter/inshorts_flutter.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'InShorts News',
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.deepPurple,
),
home: const HomePage());
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> with SingleTickerProviderStateMixin {
TabController? _controller;
List categories = [NewsType.all_news, NewsType.trending, NewsType.top_stories, NewsType.business];
@override
void initState() {
super.initState();
_controller = TabController(length: categories.length, vsync: this);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: const Text('InShorts News'),
bottom: TabBar(
controller: _controller, tabs: categories.map((e) => Tab(text: InShorts.getNewsTitle(e))).toList())),
body: TabBarView(
controller: _controller,
children:
categories.map((e) => FutureBuilder<Data>(
future: InShorts.getNews(newsType: e, language: Language.en),
builder: (context, snapshot) {
if (snapshot.hasData) {
return ListView.builder(
itemCount: snapshot.data?.newsList?.length ?? 0,
itemBuilder: (context, index) {
News news = snapshot.data!.newsList![index];
return ListTile(
leading: Image.network(news.newsObj!.imageUrl!, width: 80, fit: BoxFit.fitWidth),
title: Text(news.newsObj!.title!),
subtitle: Text(
news.newsObj!.sourceName!,
style: const TextStyle(color: Colors.grey, fontWeight: FontWeight.bold, fontSize: 13),
),
);
},
);
} else if (snapshot.hasError) {
return const Center(child: Text('Errr'));
}
return const Center(child: CircularProgressIndicator());
})).toList()
,
),
);
}
@override
void dispose() {
_controller?.dispose();
super.dispose();
}
}
Data
class consists of following,
class Data{
List<News>? newsList;
String? message;
}
News
class consists of following,
class News{
String? hashId;
int? rank;
int? version;
String? type;
bool? readOverride;
bool? fixedRank;
NewsObj? newsObj;
}
NewsObj
class consists of following,
class NewsObj{
String? oldHashId;
String? hashId;
String? authorName;
String? content;
String? sourceUrl;
String? sourceName;
String? title;
bool? important;
String? imageUrl;
String? shortenedUrl;
int? createdAt;
int? score;
List<String>? categoryNames;
List<String>? relevancyTags;
String? tenant;
String? fbObjectId;
int? fbLikeCount;
String? countryCode;
List<String>? targetedCity;
String? bottomHeadline;
String? bottomText;
bool? darkerFonts;
String? bottomPanelLink;
String? bottomType;
int? version;
bool? dontShowAd;
String? pollTenant;
bool? videoOpinionEnabled;
String? language;
bool? showInshortsBrandName;
bool? imageForRepresentation;
}
Make use of NewsObj
class to access news headline, content, image, source and more.
✍️ Authors
- Ashish Pipaliya - Author
📜 License
This project is licensed under the MIT License - see the LICENSE file for details.
🧰 Contribution
Feel free to raise issues and open PR