Flutter Switch Clipper
A Flutter package that two widgets switch with clipper.
体验一下
体验网址:Demo
使用
使用FillClipper并自定义相关参数
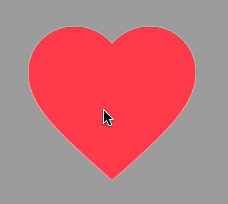
View code
SwitchClipper(
initSelect: true,
child: const Icon(Icons.favorite, size: 200, color: Colors.redAccent),
background: const Icon(Icons.favorite, size: 200, color: Colors.white),
duration: const Duration(milliseconds: 800),
customClipperBuilder: (Animation<double> animation) => FillClipper(
animation: animation,
fillAlignment: _alignment,
fillOffset: 50,
),
),
使用默认FillClipper
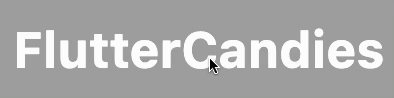
View code
const SwitchClipper(
enableWhenAnimating: false,
child: Text(
'FlutterCandies',
style: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 50,
color: Colors.amber,
height: 2,
),
),
background: Text(
'FlutterCandies',
style: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 50,
color: Colors.white,
height: 2,
),
),
curve: Curves.slowMiddle,
reverseCurve: Curves.linear,
),
使用CircleClipper切换图标颜色
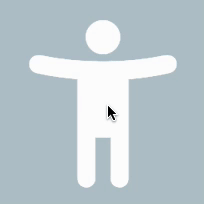
View code
SwitchClipper(
child: const Icon(Icons.accessibility_new_rounded, size: 200, color: Colors.blueAccent),
background: const Icon(Icons.accessibility_new_rounded, size: 200, color: Colors.white),
curve: Curves.ease,
duration: const Duration(milliseconds: 800),
customClipperBuilder: (Animation<double> animation) => CircleClipper(animation: animation),
),
使用CircleClipper切换两个图标
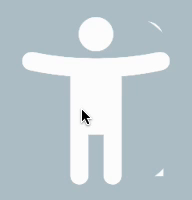
View code
SwitchClipper(
child: ColoredBox(
color: Colors.blueGrey[200] ?? Colors.blueGrey,
child: const Icon(Icons.accessibility_new_rounded, size: 200, color: Colors.white)),
background: const Icon(Icons.accessible_forward_outlined, size: 200, color: Colors.white),
curve: Curves.ease,
duration: const Duration(milliseconds: 800),
customClipperBuilder: (Animation<double> animation) => CircleClipper(animation: animation),
),
使用ShutterClipper
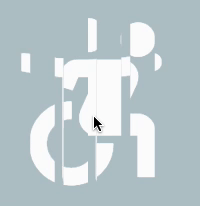
View code
SwitchClipper(
child: ColoredBox(
color: Colors.blueGrey[200] ?? Colors.blueGrey,
child: const Icon(Icons.accessibility_new_rounded, size: 200, color: Colors.white)),
background: const Icon(Icons.accessible_forward_outlined, size: 200, color: Colors.white),
curve: Curves.ease,
duration: const Duration(milliseconds: 800),
customClipperBuilder: (Animation<double> animation) => ShutterClipper(
animation: animation,
activeAlignment: _alignment,
),
),
使用WaveClipper
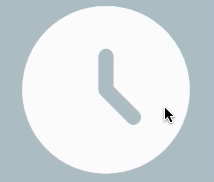
View code
SwitchClipper(
child: const Icon(Icons.access_time_filled_rounded, size: 200, color: Colors.blue),
background: const Icon(Icons.access_time_filled_rounded, size: 200, color: Colors.white),
curve: Curves.ease,
duration: const Duration(milliseconds: 2000),
customClipperBuilder: (Animation<double> animation) => WaveClipper(
animation: animation,
waveAlignment: _alignment == FillAlignment.left ? WaveAlignment.left : WaveAlignment.right,
),
),
使用CameraClipper
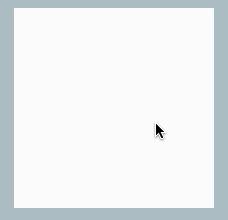
View code
SwitchClipper(
child: Container(
width: 200,
height: 200,
alignment: Alignment.center,
decoration: const BoxDecoration(
shape: BoxShape.circle,
color: Colors.blue,
),
child: const Text(
'Camera',
style: TextStyle(color: Colors.white, fontSize: 40, fontWeight: FontWeight.bold),
),
),
background: Container(
width: 200,
height: 200,
decoration: const BoxDecoration(
shape: BoxShape.circle,
color: Colors.white,
),
),
duration: const Duration(milliseconds: 2000),
customClipperBuilder: (Animation<double> animation) => CameraClipper(
animation: animation,
),
),