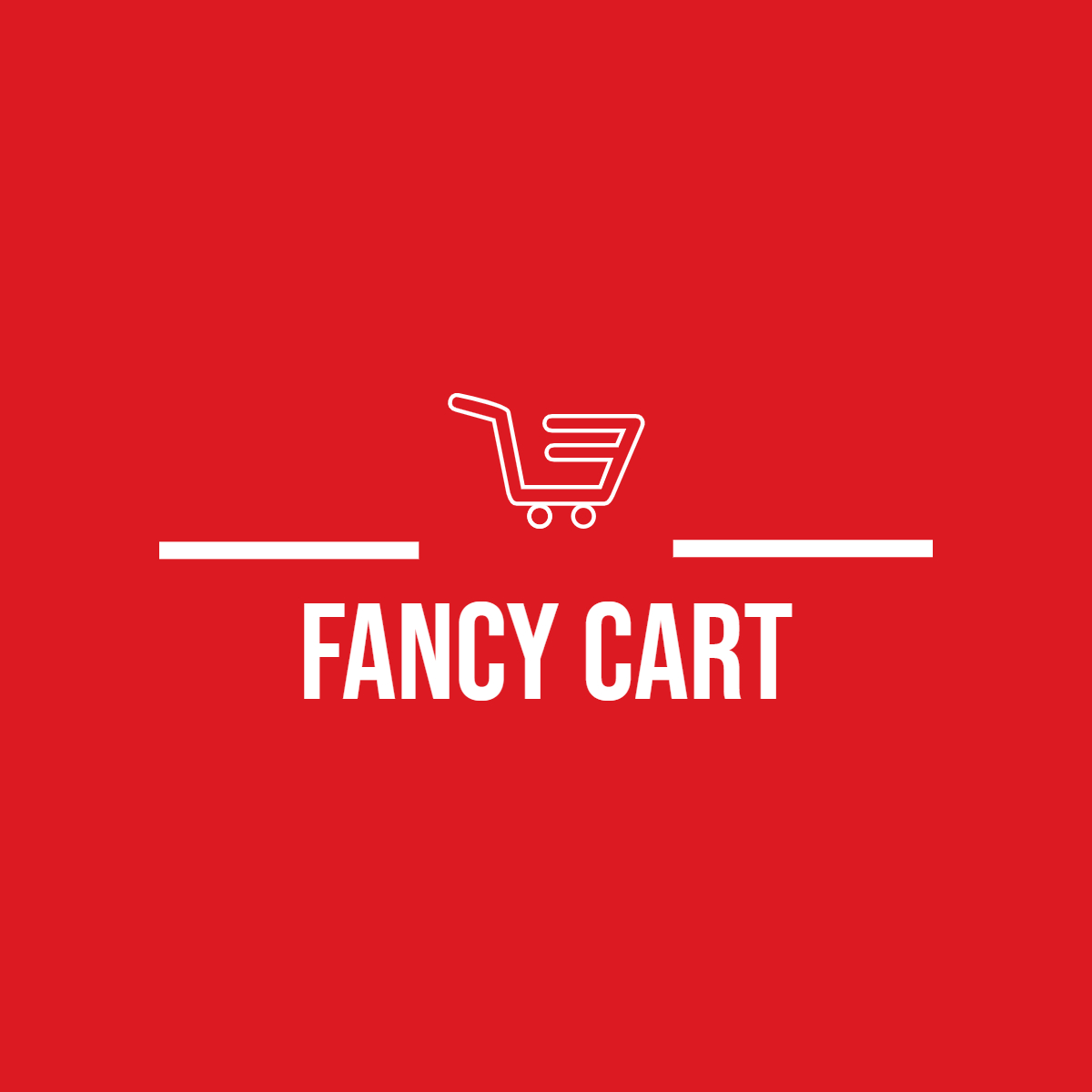
Fancy Cart Package
Welcome to the Fancy Cart package this package depend on:
1: Hive Local Storage
2: Riverpod State Management
so before we start , if you dont know more about Hive and Riverpod , recommend you to visit this website Hive and riverpod
Table of content
1: Features
2: How it works
3: Explain each method inside notifier
4: Example how to implement it
5: Types of Cart
Features
1: add items inside cart
2: remove item from cart
3: clear cart list
4: increment and decrement numer of quantities
5: get total price for cart
6: get total items inside cart
7: types of fancy cart pages
How it Works
First thing we need what data can cart item need to hold
@HiveField(0)
final int id;
@HiveField(1)
final String name;
@HiveField(2)
final String image;
@HiveField(3)
double price;
@HiveField(4)
int quantity;
@HiveField(5)
final Map<String, dynamic> additionalData;
Initialize
init hive and register cart item adapter then open cart box to store cart items,
as its depend on hive so we need to initialize it in main.dart
static initialize() async {
await Hive.initFlutter();
Hive.registerAdapter(CartItemAdapter());
await Hive.openBox<CartItem>('cart');
}
Cart List
init cart list inside notifier
List<CartItem> _cartList = [];
List<CartItem> get cartList => _cartList;
CartNotifier() {
fetchCart();
}
Add Item to Cart
if item already exist in cart then update its quantity
else add it to cart.
Future<void> addItem(CartItem cart) async {
}
Remove Item from Cart
Future<void> removeItem(CartItem cart) async {
}
Fetch items
Future<void> fetchCart() async {
}
Clear Cart
Future<void> clearCart() async {
}
Increment qunantity
have an option to update price for item when increment
Future<void> incrementItemQuantity(CartItem cart) async {
}
Decrement quantity
there is 2 behaviors here:
1: if deleteOption
is true then delete item from cart if quantity is less than 1
2: else if deleteOption
is false then it doesn't delete item from cart if quantity is less than 1
3: else decrement item quantity and update its price
there is an option actionAfterDelete
to make action after delete item like showing snackBar, etc.
there is an option notDecrementedAction
to make action if item quantity is less than 1 and deleteOption
is false like showing snackBar, etc.
Future<void> decrementItemQuantity(CartItem cart, {bool deleteOption = false,Function? actionAfterDelete, Function? notDecrementedAction}) async {
}
get total price in cart
double getTotalPrice() {
}
get price for each item
you have an option updatePrice
to update item price in cart,
if you set it to true then it will update item price in cart by multiplying item price by item quantity
else it will return item price without updating it
double getPriceForItem(CartItem cart, {bool updatePrice = false}) {
}
get total items in cart
if needToIncludeQuantity
is true then it will return total number of items in cart including quantity
else it will return number of items in cart
int getNumberOfItemsInCart({bool needToIncludeQuantity = true}) {
}
Example
how to implement it inside your project
init
init cart notifier inside main
void main() async {
WidgetsFlutterBinding.ensureInitialized();
/// initialize fancy cart
initializeFancyCart(
child: const MyApp(),
);
}
Example
import 'dart:developer';
import 'package:fancy_cart/fancy_cart.dart';
import 'package:flutter/material.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
// initialize fancy cart
initializeFancyCart(
child: const MyApp(),
);
}
class MyApp extends StatefulWidget {
const MyApp({Key? key}) : super(key: key);
@override
State<MyApp> createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
/// Example of using Fancy Cart
home: Scaffold(
appBar: AppBar(
title: const Text('Test Cart'),
actions: [
// ----------------- Clear Cart ----------------- //
/// clear cart button with action after delete
ClearCartButton(
child: const Icon(Icons.delete),
actionAfterDelete: () {
log("cart deleted", name: "cart deleted");
},
),
// ----------------- Total Items in Cart ----------------- //
/// total items in cart, with option to include quantity or not (default is true)
TotalItemsCartWidget(totalItemsBuilder: (totalItems) {
return Text(totalItems.toString());
})
],
),
// ----------------- Cart Widget ----------------- //
/// cart widget with custom builder for cart list which contains controller to add, remove, clear cart
body: CartWidget(
cartBuilder: (controller) {
return Column(
children: [
Expanded(
child: ListView.builder(
itemCount: controller.cartList.length,
itemBuilder: (context, index) {
final cartItem = controller.cartList[index];
return ListTile(
title: Text(cartItem.name),
subtitle: Text(controller
.getPriceForItem(cartItem, updatePrice: true)
.toString()),
trailing: Row(
mainAxisSize: MainAxisSize.min,
children: [
IconButton(
onPressed: () {
controller.incrementItemQuantity(cartItem);
},
icon: const Icon(Icons.add),
),
Text(cartItem.quantity.toString()),
IconButton(
onPressed: () {
controller.decrementItemQuantity(cartItem);
},
icon: const Icon(Icons.remove),
),
],
),
leading: IconButton(
icon: const Icon(Icons.remove),
onPressed: () {
controller.removeItem(cartItem);
},
),
);
},
),
),
// ----------------- add to cart button ----------------- //
/// add to cart button with action after add and model to add
AddToCartButton(
actionAfterAdding: () {
log("item added", name: "item added");
},
cartModel: CartItem(
id: DateTime.now().millisecondsSinceEpoch,
name: 'Test',
price: 100,
image: ""),
child: Container(
height: 50,
margin: const EdgeInsets.all(10),
width: double.infinity,
decoration: BoxDecoration(
color: Colors.blue,
borderRadius: BorderRadius.circular(10),
),
child: const Center(
child: Text(
"Add to cart",
style: TextStyle(color: Colors.white),
),
),
),
),
// ----------------- Total price ----------------- //
/// total price of cart
Text("Total Price : ${controller.getTotalPrice()}"),
],
);
},
),
));
}
}
Types Of Cart
Trolley Cart
class TrolleyCartScreen extends StatelessWidget {
const TrolleyCartScreen({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.grey[200],
body: SafeArea(
child: Padding(
padding: const EdgeInsets.only(top: 15),
child: Column(
children: [
const TrolleyHeader(),
const SizedBox(
height: 10,
),
const ShoppingCartTrolley(quantityCardDirection: Direction.vertical),
const SizedBox(
height: 10,
),
TrolleyCheckOut(
shippingFee: 10,
onCheckout: () {},
),
],
),
)),
);
}
}
Modal Cart
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: AddToCartButton(
actionAfterAdding: () {
BottomSheetCart.show(
context: context,
checkOutButton: (cartList){
log(cartList.length.toString());
},
checkOutPaypalButton: (cartList){
log(cartList.length.toString());
}
);
},
cartModel: CartModel(
id: 9998,
name: 'Isabella Chair',
price: 250.00,
image:
"https://encrypted-tbn0.gstatic.com/images?q=tbn:ANd9GcRASO0ZI6-YXskH0epPedmjRp-Ks01bfGobuYQ-pirFNjg0QOwF37pV_MHQA4bcGn3dMHk&usqp=CAU",
additionalData: {
"color": "pink",
},
),
child: Container(
height: 50,
margin: const EdgeInsets.all(10),
width: double.infinity,
decoration: BoxDecoration(
color: Colors.blue,
borderRadius: BorderRadius.circular(10),
),
child: const Center(
child: Text(
"Add to cart",
style: TextStyle(color: Colors.white),
),
),
),
),
),
);
}
}