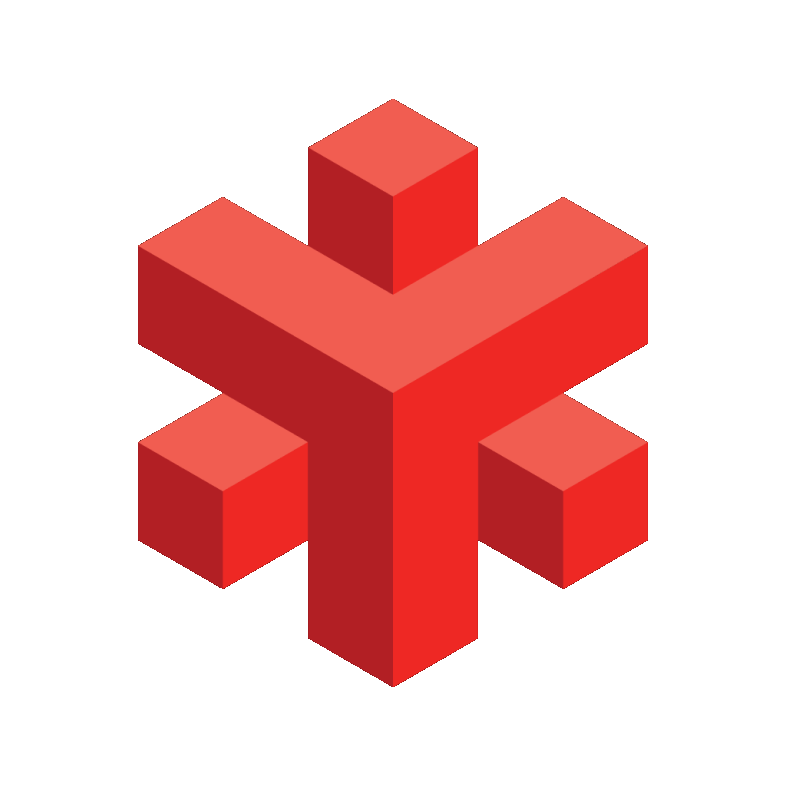
🌟 DjangoFlow OAuth Flutter Package 🌟
djangoflow_oauth makes OAuth2 flows easy for Flutter apps! This package supports secure PKCE-based OAuth2 authentication with multiple providers, including Google, Facebook, and custom OAuth servers. Works seamlessly across mobile (Android/iOS) and web platforms with minimal configuration. 🚀
🚀 Features 🚀
- PKCE Flow: Secure OAuth2 with Proof Key for Code Exchange.
- Web and Mobile Support: Works on Android, iOS, and Web.
- Multiple Providers: Add any OAuth2 provider with minimal setup.
- Based on Flutter Best Practices: Easy-to-use, extendable, and DRY code.
📑 Table of Contents 📑
📦 Installation 📦
Add the djangoflow_oauth
package to your pubspec.yaml
:
dependencies:
djangoflow_oauth: <latest_version>
Run the following command to install the package:
flutter pub get
🔧 Setup 🔧
To use the djangoflow_oauth
package, create your custom OAuth provider by implementing the OAuthProvider
interface.
import 'package:djangoflow_oauth/djangoflow_oauth.dart';
class MyOAuthProvider implements OAuthProvider {
@override
final String clientId = 'your-client-id';
@override
final String authorizationEndpoint = 'https://example.com/auth';
@override
final String tokenEndpoint = 'https://example.com/token';
@override
final String redirectUrl = 'com.example.app:/oauth2redirect';
@override
final List<String> scopes = ['email', 'profile'];
}
💡 Usage 💡
Initializing OAuth Provider
final provider = MyOAuthProvider();
final pkceFlow = PKCEFlow(provider);
Authorizing Users
Use the authorize
method to start the OAuth2 flow.
try {
final code = await pkceFlow.authorize();
print('Authorization Code: $code');
} catch (e) {
print('Authorization failed: $e');
}
Exchanging Auth Code for Token
After obtaining the authorization code, exchange it for an access token.
try {
final tokenResponse = await pkceFlow.exchangeAuthCodeForToken({});
print('Access Token: ${tokenResponse?['access_token']}');
} catch (e) {
print('Token exchange failed: $e');
}
🌟 Example 🌟
Check out the example for a complete implementation.
📄 License 📄
This package is distributed under the MIT License.