Dart Connect Metro
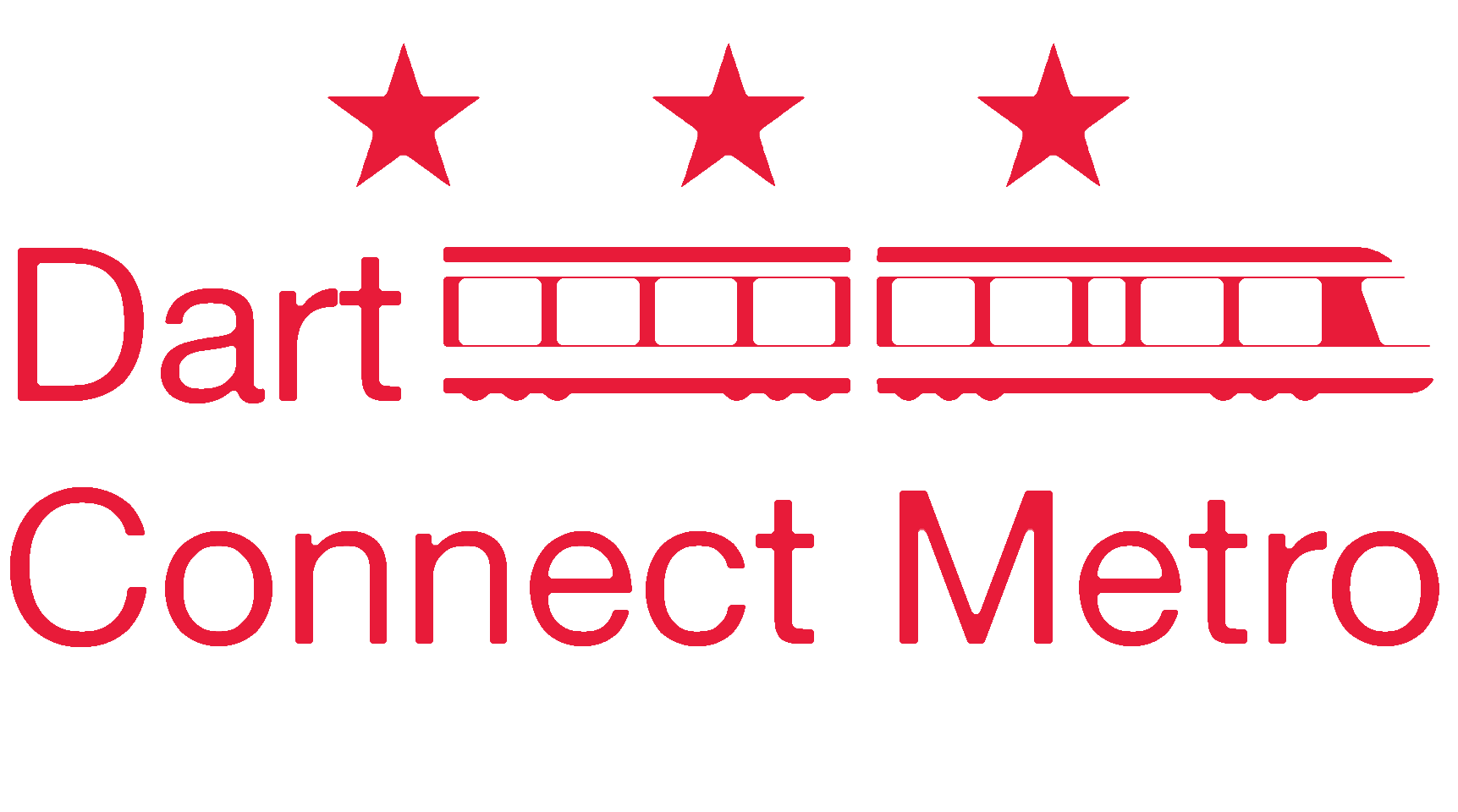
Dart Connect Metro is a valuable resource for developers looking to integrate data from the Washington DC Metro system into their applications. This SDK provides convenient access to essential information, including real-time updates and schedules, allowing developers to enhance the functionality and user experience of their DC Metro-related apps.
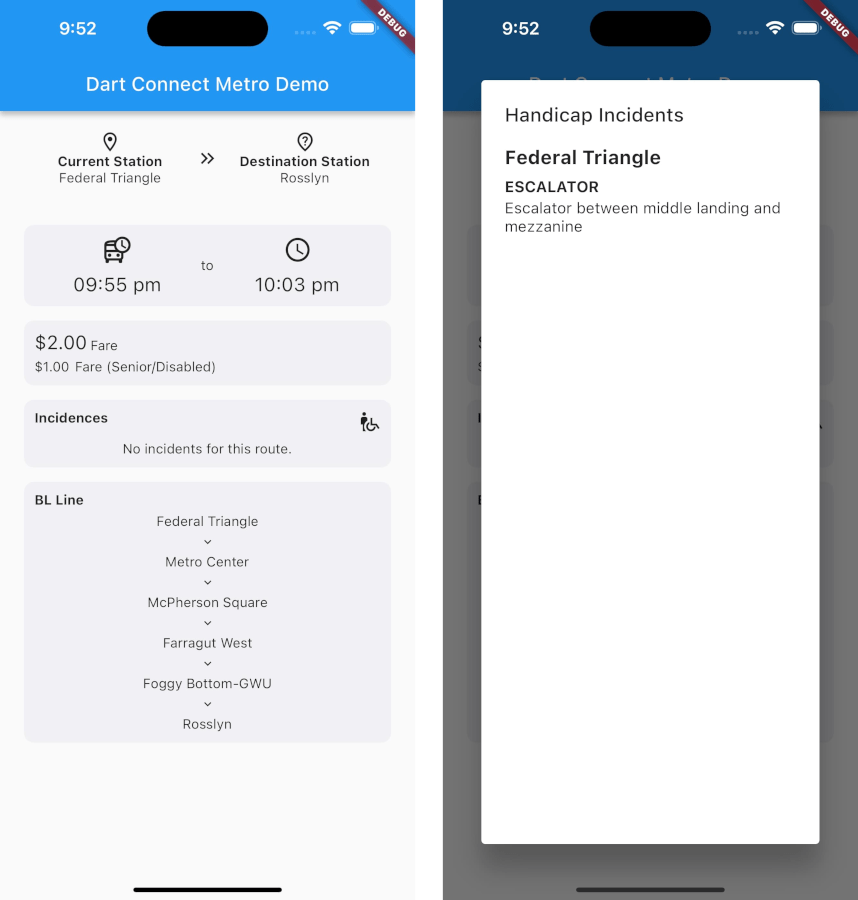
Installation
In the pubspec.yaml
of your project, add the following dependency:
dependencies:
dart_connect_metro: ^0.1.2
Import it to each file you use it in:
import 'package:dart_connect_metro/dart_connect_metro.dart';
Getting Started
Generate Your API Keys
You will need to generate custom API keys for your program on the Washington Metropolitan Area Transit Authority (WMATA) website.
First, create an account here.
Next, go to the products page and subscribe (the default tier is free).
Finally, go to your profile page to see your API keys.
Usage
Example 1 - Trip Cost
This example shows how to get the cost of a trip. There is some nuance to be aware of; there is a normal fare and a senior/disabled citizen fare. The normal fare is further broken down into peak/non-peak hours.
// The station codes for the example route. Normally, you would get these
// programmatically.
static const String federalTriangleCode = 'D01';
static const String rosslynCode = 'C05';
/// Fetch the station to station information.
List<StationToStation> stationToStation =
await fetchStationToStation(
apiKey,
startStationCode: federalTriangleCode,
destinationStationCode: rosslynCode,
);
/// Get the cost of the trip.
final double cost = stationToStation.first.railFare.currentFare;
/// Get the cost of the trip for a senior citizen.
final double seniorCost = stationToStation.first.railFare.seniorCitizen;
Example 2 - Stations on Path
This example shows how to get the names of all the stations on a route.
/// Fetch the path information.
Path path = await fetchPath(
apiKey,
startStationCode: federalTriangleCode,
destinationStationCode: rosslynCode,
);
List<String> stationNames = [];
for(Path pathItem in path.items) {
stationNames.add(pathItem.stationName);
}
Example 3 - Departure and Arrival Times
This example shows how to find the next departure and arrival times for a route going from one station to another.
/// Fetch the station to station information.
List<StationToStation> stationToStation =
await fetchStationToStation(
apiKey,
startStationCode: federalTriangleCode,
destinationStationCode: rosslynCode,
);
/// Fetch the path information.
Path path = await fetchPath(
apiKey,
startStationCode: federalTriangleCode,
destinationStationCode: rosslynCode,
);
/// Fetch the next train information.
List<NextTrain> nextTrain = await fetchNextTrains(
apiKey,
stationCodes: [federalTriangleCode],
);
// Get line code to match with next train.
final String lineCode = path.items.first.lineCode;
// Get the next train for the line.
late final NextTrain nextTrain;
for (NextTrain train in nextTrains) {
if (train.line == lineCode) {
nextTrain = train;
break;
}
}
// Get the time of the next train.
final DateTime nextTrainTime =
DateTime.now().add(Duration(minutes: nextTrain.minutesAway ?? 0));
/// The time when the train will arrive to pick you up to start your trip.
final Time departureTime = Time.fromDateTime(nextTrainTime);
/// The time when you will arrive at your destination station.
final Time arrivalTime = Time.fromDateTime(nextTrainTime);
// Add the minutes of the journey to get arrival time.
for (StationToStation station in stationToStation) {
arrivalTime.add(Duration(minutes: station.travelTimeMinutes));
}
For more examples, view the example project that comes in this package.
If you found this helpful, please consider donating. Thanks!
Libraries
- constants/api_fields
- dart_connect_metro
- features/bus_predictions/domain/models/next_buses/next_buses
- features/bus_predictions/domain/models/next_buses/prediction
- features/bus_predictions/domain/repos/next_buses_repo
- features/bus_predictions/domain/services/next_buses_service
- features/bus_routes/domain/models/bus_position
- features/bus_routes/domain/models/path_details/path_details
- features/bus_routes/domain/models/path_details/path_details_direction
- features/bus_routes/domain/models/path_details/shape_point
- features/bus_routes/domain/models/route
- features/bus_routes/domain/models/schedule/schedule
- features/bus_routes/domain/models/schedule/schedule_direction
- features/bus_routes/domain/models/schedule/schedule_stop
- features/bus_routes/domain/models/schedule_at_stop/schedule_arrival
- features/bus_routes/domain/models/schedule_at_stop/schedule_at_stop
- features/bus_routes/domain/models/stop
- features/bus_routes/domain/repos/bus_position_repo
- features/bus_routes/domain/repos/path_details_repo
- features/bus_routes/domain/repos/routes_repo
- features/bus_routes/domain/repos/schedule_at_stop_repo
- features/bus_routes/domain/repos/schedule_repo
- features/bus_routes/domain/repos/stop_search_repo
- features/bus_routes/domain/services/bus_position_service
- features/bus_routes/domain/services/path_details_service
- features/bus_routes/domain/services/routes_service
- features/bus_routes/domain/services/schedule_at_stop_service
- features/bus_routes/domain/services/schedule_service
- features/bus_routes/domain/services/stop_search_service
- features/incidents/domain/models/ada_incident
- features/incidents/domain/models/bus_incident
- features/incidents/domain/models/rail_incident
- features/incidents/domain/repos/ada_outages_repo
- features/incidents/domain/repos/bus_incident_repo
- features/incidents/domain/repos/rail_incident_repo
- features/incidents/domain/services/ada_outages_service
- features/incidents/domain/services/bus_incident_service
- features/incidents/domain/services/rail_incident_service
- features/misc/domain/repos/validate_api_key_repo
- features/misc/domain/services/validate_api_key_service
- features/rail_predictions/domain/models/next_train
- features/rail_predictions/domain/repos/next_trains_repo
- features/rail_predictions/domain/services/next_trains_service
- features/rail_station_info/domain/models/line
- features/rail_station_info/domain/models/parking/all_day_parking
- features/rail_station_info/domain/models/parking/parking
- features/rail_station_info/domain/models/parking/short_term_parking
- features/rail_station_info/domain/models/path/path
- features/rail_station_info/domain/models/path/path_item
- features/rail_station_info/domain/models/station/address
- features/rail_station_info/domain/models/station/station
- features/rail_station_info/domain/models/station_entrance
- features/rail_station_info/domain/models/station_timings/day_timings
- features/rail_station_info/domain/models/station_timings/station_timings
- features/rail_station_info/domain/models/station_timings/terminus_train
- features/rail_station_info/domain/models/station_to_station/rail_fare
- features/rail_station_info/domain/models/station_to_station/station_to_station
- features/rail_station_info/domain/repos/lines_repo
- features/rail_station_info/domain/repos/parking_repo
- features/rail_station_info/domain/repos/path_between_stations_repo
- features/rail_station_info/domain/repos/station_entrances_repo
- features/rail_station_info/domain/repos/station_repo
- features/rail_station_info/domain/repos/station_timings_repo
- features/rail_station_info/domain/repos/station_to_station_repo
- features/rail_station_info/domain/services/lines_service
- features/rail_station_info/domain/services/parking_service
- features/rail_station_info/domain/services/path_between_stations_service
- features/rail_station_info/domain/services/station_entrances_service
- features/rail_station_info/domain/services/station_service
- features/rail_station_info/domain/services/station_timings_service
- features/rail_station_info/domain/services/station_to_station_service
- features/train_positions/domain/models/service_type
- features/train_positions/domain/models/standard_routes/route_track_circuit
- features/train_positions/domain/models/standard_routes/standard_route
- features/train_positions/domain/models/track_circuits/track_circuit
- features/train_positions/domain/models/track_circuits/track_circuit_neighbor
- features/train_positions/domain/models/train_position
- features/train_positions/domain/repos/standard_route_repo
- features/train_positions/domain/repos/track_circuits_repo
- features/train_positions/domain/repos/train_position_repo
- features/train_positions/domain/services/standard_route_service
- features/train_positions/domain/services/track_circuits_service
- features/train_positions/domain/services/train_position_service
- utils/coordinate_calculator
- utils/date_time_formatter
- utils/json_tool
- utils/time