Country picker
A flutter package to select a country from a list of countries.
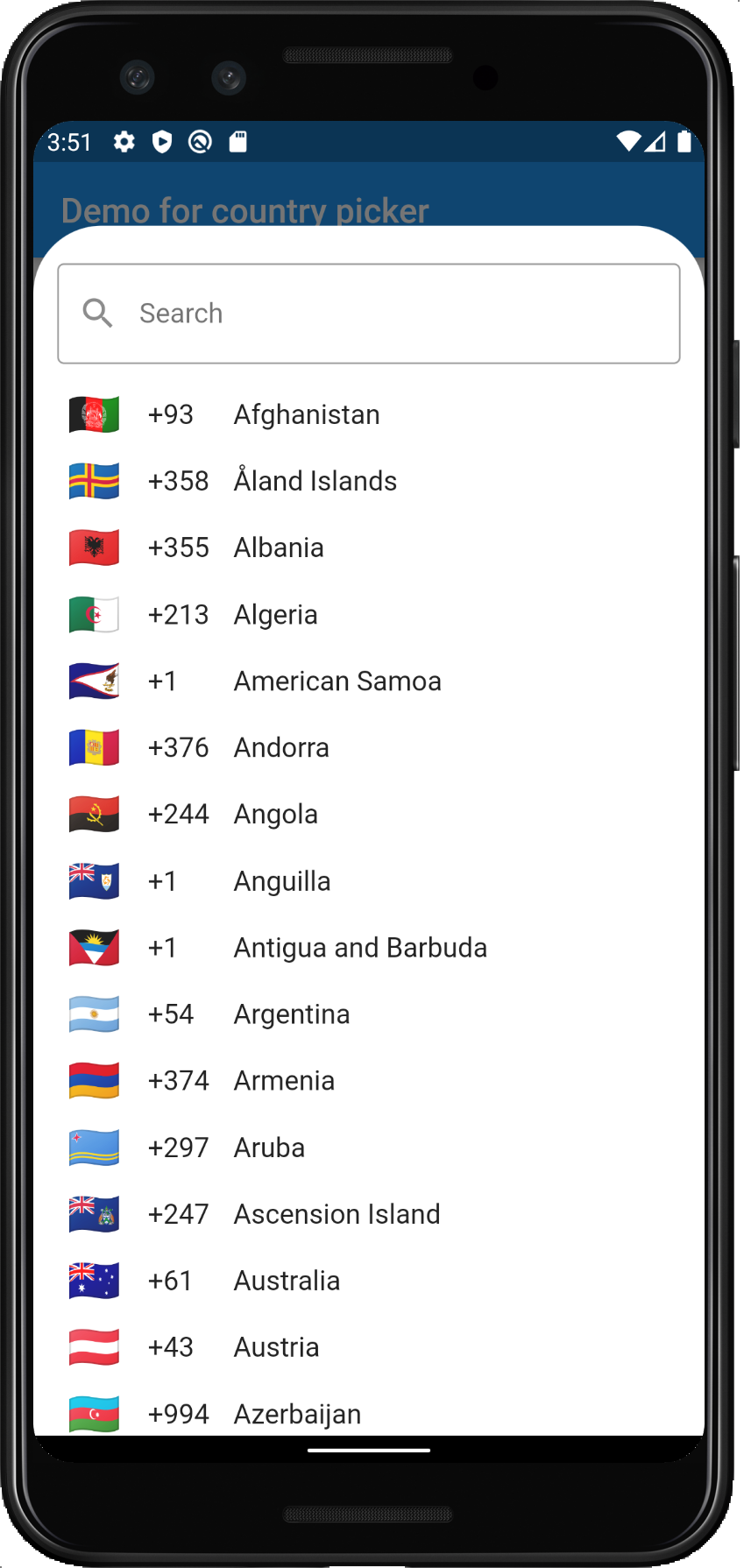
Getting Started
Add the package to your pubspec.yaml:
country_picker: ^2.0.27
In your dart file, import the library:
import 'package:country_picker/country_picker.dart';
Show country picker using showCountryPicker
:
showCountryPicker(
context: context,
showPhoneCode: true, // optional. Shows phone code before the country name.
onSelect: (Country country) {
print('Select country: ${country.displayName}');
},
);
For localization:
Add the CountryLocalizations.delegate
in the list of your app delegates.
MaterialApp(
supportedLocales: [
const Locale('en'),
const Locale('el'),
const Locale.fromSubtags(languageCode: 'zh', scriptCode: 'Hans'), // Generic Simplified Chinese 'zh_Hans'
const Locale.fromSubtags(languageCode: 'zh', scriptCode: 'Hant'), // Generic traditional Chinese 'zh_Hant'
],
localizationsDelegates: [
CountryLocalizations.delegate,
GlobalMaterialLocalizations.delegate,
GlobalWidgetsLocalizations.delegate,
GlobalCupertinoLocalizations.delegate,
],
home: HomePage(),
);
Parameters:
onSelect
: Called when a country is selected. The country picker passes the new value to the callback (required)onClosed
: Called when CountryPicker is dismissed, whether a country is selected or not (optional).showPhoneCode
: Can be used to show phone code before the country name.searchAutofocus
Can be used to initially expand virtual keyboardshowSearch
Can be used to show/hide the search bar.showWorldWide
An optional argument for showing "World Wide" option at the beginning of the listfavorite
Can be used to show the favorite countries at the top of the list (optional).moveAlongWithKeyboard
Can be used to move bottomSheet along with keyboard when textfield is focused (optional).countryListTheme
: Can be used to customize the country list's bottom sheet and widgets that lie within it. (optional).showCountryPicker( context: context, countryListTheme: CountryListThemeData( flagSize: 25, backgroundColor: Colors.white, textStyle: TextStyle(fontSize: 16, color: Colors.blueGrey), bottomSheetHeight: 500, // Optional. Country list modal height //Optional. Sets the border radius for the bottomsheet. borderRadius: BorderRadius.only( topLeft: Radius.circular(20.0), topRight: Radius.circular(20.0), ), //Optional. Styles the search field. inputDecoration: InputDecoration( labelText: 'Search', hintText: 'Start typing to search', prefixIcon: const Icon(Icons.search), border: OutlineInputBorder( borderSide: BorderSide( color: const Color(0xFF8C98A8).withOpacity(0.2), ), ), ), ), onSelect: (Country country) => print('Select country: ${country.displayName}'), );
exclude
: Can be used to exclude(remove) one or more country from the countries list (optional).showCountryPicker( context: context, exclude: <String>['KN', 'MF'], //It takes a list of country code(iso2). onSelect: (Country country) => print('Select country: ${country.displayName}'), );
countryFilter
: Can be used to filter the countries list (optional).- It takes a list of country code(iso2).
- Can't provide both exclude and countryFilter
Contributions
Contributions of any kind are more than welcome! Feel free to fork and improve country_code_picker in any way you want, make a pull request, or open an issue.