Analytics
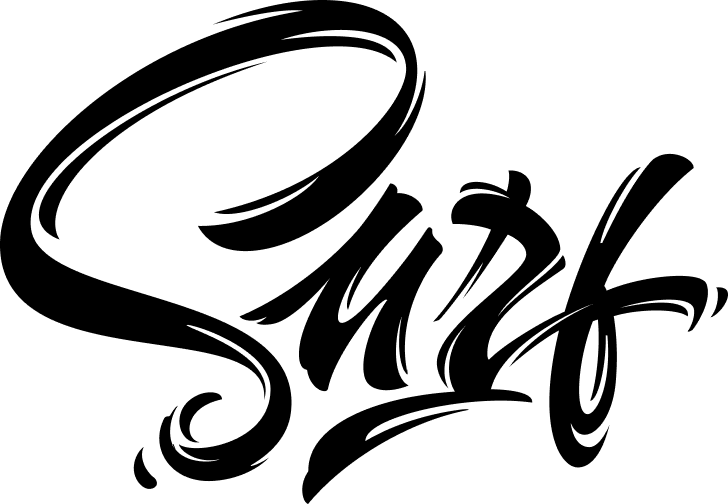
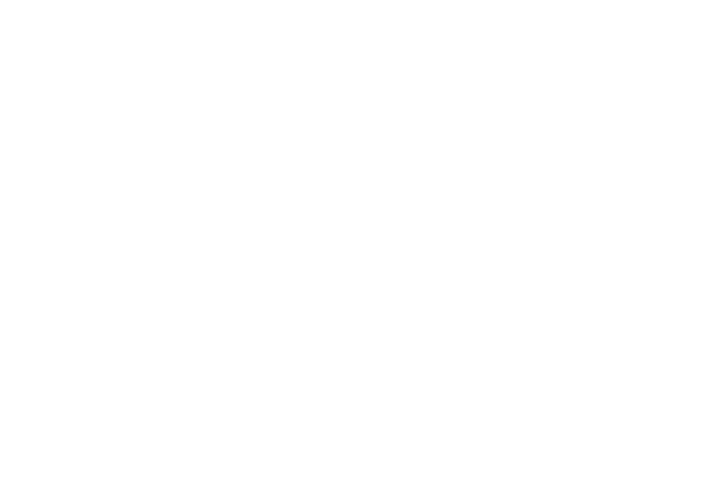
Made by Surf 🏄♂️🏄♂️🏄♂️
Overview
Interface for working with analytic services. The library is supposed to unify work with various analytic services. The main actors are:
- AnalyticAction — any action that is valuable for analytics. Usually it is a "button pressed" or "screen opened" type of event but the main criterion is a possibility to be handled by
AnalyticStrategy
. - AnalyticActionPerformer - an interface for analytic action performers. This class is an abstract base class for analytic strategies.
- AnalyticStrategy — a class that provides a special way to send an analytical action. To create a custom analytic strategy, you should extend this class and implement the
performAction
method. - AnalyticService — a class that provides a way to send analytic action using a set of analytic strategies. To use this class, you should create an instance of it with a set of analytic strategies, and then call the
performAction
method to send analytic action.
Example
The easiest interaction with the library is as follows:
-
Determine the actions that ought to be recorded in the analytics service:
class MyAnalyticAction implements AnalyticAction { final String key; final String value; MyAnalyticAction(this.key, this.value); } class ButtonPressedAction extends MyAnalyticAction { ButtonPressedAction() : super("button_pressed", null); } class ScreenOpenedAction extends MyAnalyticAction { ScreenOpenedAction({String param}) : super("screen_opened", param); }
-
Implement analytic strategy:
class MyAnalyticStrategy extends AnalyticStrategy<MyAnalyticAction> { final SomeAnalyticsApi _analyticsApi; SomeAnalyticsApi(this._analyticsApi); @override void performAction(MyAnalyticAction action) { _analyticsApi.send(action.key, action.value); } }
-
Create an AnalyticService with your strategy:
final analyticService = AnalyticService.withStrategies({ MyAnalyticStrategy(analytics), });
Usage:
analyticService.performAction(ButtonPressedAction());
Installation
Add analytics
to your pubspec.yaml
file:
dependencies:
analytics: $currentVersion$
At this moment, the current version of analytics
is .
Migrating from 1.x.x to 2.x.x
1.x.x
final analyticService = DefaultAnalyticService();
analyticService.addActionPerformer(
MyAnalyticActionPerformer(SomeAnalyticService()),
);
Starting from version 2.0.0, sending an event to a specific analytics service is implemented using strategies. The AnalyticService
class stores these strategies. Implement analytics strategies using the AnalyticStrategy
egy class.
final analyticService = AnalyticService.withStrategies({
MyAnalyticStrategy(analytics),
});
Changelog
All notable changes to this project will be documented in this file.
Issues
To report your issues, submit directly in the Issues section.
Contribute
If you would like to contribute to the package (e.g. by improving the documentation, fixing a bug or adding a cool new feature), please read our contribution guide first and send us your pull request.
Your PRs are always welcome.
How to reach us
Please feel free to ask any questions about this package. Join our community chat on Telegram. We speak English and Russian.